Auto Hyphenation
Hyphenation is the process of breaking words between lines to create more consistency across a text block.
GemBox.Document supports auto hyphenation while exporting to PDF, XPS, and image file formats.
The following example shows how you can control auto hyphenation using available hyphenation options.
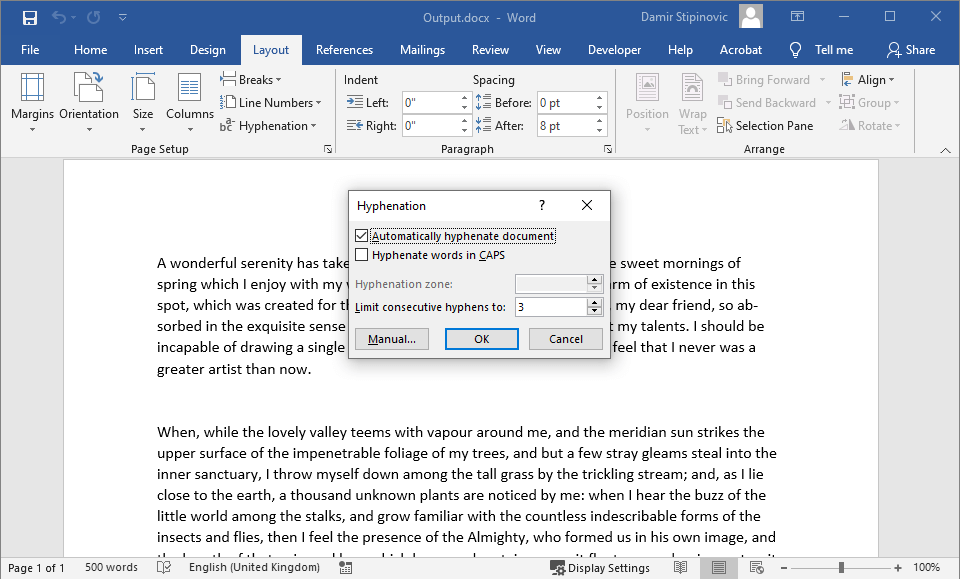
using GemBox.Document;
using GemBox.Document.Hyphenation;
using System.Globalization;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load Word document from file's path.
var document = DocumentModel.Load("%InputFileName%");
// Get Word document's hyphenation options.
var hyphenationOptions = document.HyphenationOptions;
// Enable auto hyphenation, set the maximum number of consecutive lines,
// and disable hyphenation of words in all capital letters.
hyphenationOptions.AutoHyphenation = true;
hyphenationOptions.ConsecutiveHyphensLimit = 3;
hyphenationOptions.HyphenateCaps = false;
// Save output file.
document.Save("Output.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Hyphenation
Imports System.Globalization
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load Word document from file's path.
Dim document = DocumentModel.Load("%InputFileName%")
' Get Word document's hyphenation options.
Dim hyphenationOptions = document.HyphenationOptions
' Enable auto hyphenation, set the maximum number of consecutive lines,
' and disable hyphenation of words in all capital letters.
hyphenationOptions.AutoHyphenation = True
hyphenationOptions.ConsecutiveHyphensLimit = 3
hyphenationOptions.HyphenateCaps = False
' Save output file.
document.Save("Output.%OutputFileType%")
End Sub
End Module
Register a hyphenation dictionary
In addition to the built-in English hyphenation dictionary, custom hyphenation dictionaries can be registered for other languages.
You can use any publicly available TeX based hyphenation dictionary, a .tex file containing hyphenation patterns with specific encoding.
The following example shows how you can register a custom hyphenation dictionary.
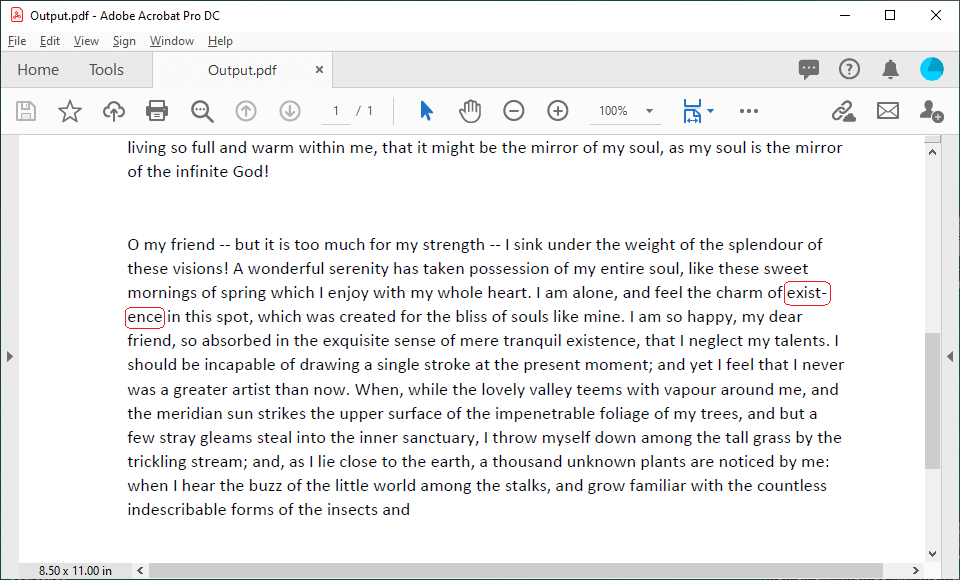
using GemBox.Document;
using GemBox.Document.Hyphenation;
using System.Globalization;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load Word document from file's path.
var document = DocumentModel.Load("%InputFileName%");
// Enable auto hyphenation.
document.HyphenationOptions.AutoHyphenation = true;
// Load hyphenation dictionary from file's path.
var hyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEnGb.tex%");
// Set loaded hyphenation dictionary for specified language.
DocumentModel.HyphenationDictionaries[new CultureInfo("en-GB")] = hyphenationDictionary;
// Save output file.
document.Save("OutputCustomHyphenation.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Hyphenation
Imports System.Globalization
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load Word document from file's path.
Dim document = DocumentModel.Load("%InputFileName%")
' Enable auto hyphenation.
document.HyphenationOptions.AutoHyphenation = True
' Load hyphenation dictionary from file's path.
Dim hyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEnGb.tex%")
' Set loaded hyphenation dictionary for specified language.
DocumentModel.HyphenationDictionaries(New CultureInfo("en-GB")) = hyphenationDictionary
' Save output file.
document.Save("OutputCustomHyphenation.%OutputFileType%")
End Sub
End Module
Hyphenation of multi-language documents
When processing multi-language documents, it can be helpful to delay the loading of hyphenation dictionaries, using the HyphenationDictionary
class.
The following example shows how you can delay the loading of hyphenation dictionaries for multi-language documents.
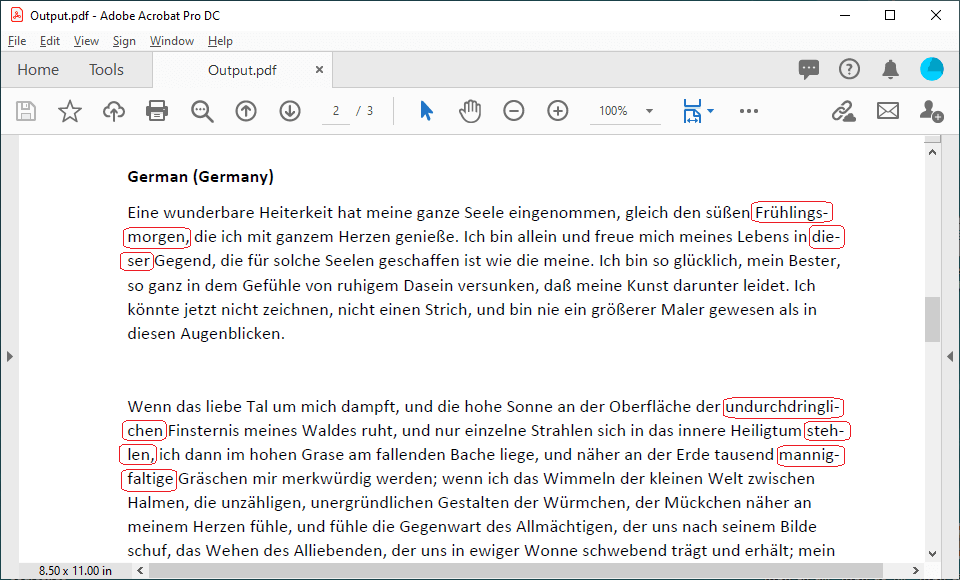
using GemBox.Document;
using GemBox.Document.Hyphenation;
using System.Globalization;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load Word document from file's path.
var document = DocumentModel.Load("%InputFileName%");
// Enable auto hyphenation.
document.HyphenationOptions.AutoHyphenation = true;
// Load hyphenation dictionaries on demand and set them for specified languages.
DocumentModel.HyphenationDictionaries.HyphenationDictionaryLoading +=
(sender, e) =>
{
switch (e.CultureInfo.Name)
{
case "en-GB":
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEnGb.tex%");
break;
case "de-DE":
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictDe.tex%");
break;
case "es-ES":
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEs.tex%");
break;
}
};
// Save output file.
document.Save("OutputMultiLanguage.%OutputFileType%");
}
}
Imports GemBox.Document
Imports GemBox.Document.Hyphenation
Imports System.Globalization
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load Word document from file's path.
Dim document = DocumentModel.Load("%InputFileName%")
' Enable auto hyphenation.
document.HyphenationOptions.AutoHyphenation = True
' Load hyphenation dictionaries on demand and set them for specified languages.
AddHandler DocumentModel.HyphenationDictionaries.HyphenationDictionaryLoading,
Sub(sender, e)
Select Case e.CultureInfo.Name
Case "en-GB"
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEnGb.tex%")
Exit Select
Case "de-DE"
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictDe.tex%")
Exit Select
Case "es-ES"
e.HyphenationDictionary = TexHyphenationDictionary.Load("%#HyphDictEs.tex%")
Exit Select
End Select
End Sub
' Save output file.
document.Save("OutputMultiLanguage.%OutputFileType%")
End Sub
End Module