Create Word Form Controls
The following example shows how you can create a legacy form with text, check-box, and drop-down form fields, using the GemBox.Document component.
using GemBox.Document;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = new DocumentModel();
// Create document with labels and form fields.
document.Sections.Add(
new Section(document,
new Paragraph(document,
new Run(document, "Full name: "),
new Field(document, FieldType.FormText, null, new string('\x2002', 5))
{ FormData = { Name = "FullName" } }),
new Paragraph(document,
new Run(document, "Birth date: "),
new Field(document, FieldType.FormText, null, "dd/mm/yyyy")
{ FormData = { Name = "BirthDate" } }),
new Paragraph(document,
new Run(document, "Married: "),
new Field(document, FieldType.FormCheckBox)
{ FormData = { Name = "Married" } }),
new Paragraph(document,
new Run(document, "Gender: "),
new Field(document, FieldType.FormDropDown)
{ FormData = { Name = "Gender" } })));
// Customize form fields.
var formFieldsData = document.Content.FormFieldsData;
var fullNameFieldData = (FormTextData)formFieldsData["FullName"];
fullNameFieldData.MaximumLength = 50;
fullNameFieldData.ValueFormat = "Title case";
var birthDateFieldData = (FormTextData)formFieldsData["BirthDate"];
birthDateFieldData.TextType = FormTextType.Date;
birthDateFieldData.ValueFormat = "dd/MM/yyyy";
var marriedFieldData = (FormCheckBoxData)formFieldsData["Married"];
// Status text is shown on status bar, a bottom-right corner of Microsoft Word.
marriedFieldData.StatusText = "Check if you're married.";
// Help text is shown on field when in focus and F1 is pressed.
marriedFieldData.HelpText = "Check if you're married.";
var genderFieldData = (FormDropDownData)formFieldsData["Gender"];
// First item as a default option for non-selected value.
genderFieldData.Items.Add("<Select Gender>");
genderFieldData.Items.Add("Male");
genderFieldData.Items.Add("Female");
// Make document a form by restricting editing to filling-in form fields.
document.Protection.StartEnforcingProtection(EditingRestrictionType.FillingForms, "pass");
document.Save("Create Form.%OutputFileType%");
}
}
Imports GemBox.Document
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document As New DocumentModel()
' Create document with labels and form fields.
Dim fullNameField As New Field(document, FieldType.FormText, Nothing, New String(ChrW(&H2002), 5))
fullNameField.FormData.Name = "FullName"
Dim birthDateField As New Field(document, FieldType.FormText, Nothing, "dd/mm/yyyy")
birthDateField.FormData.Name = "BirthDate"
Dim marriedField As New Field(document, FieldType.FormCheckBox)
marriedField.FormData.Name = "Married"
Dim genderField As New Field(document, FieldType.FormDropDown)
genderField.FormData.Name = "Gender"
document.Sections.Add(
New Section(document,
New Paragraph(document, New Run(document, "Full name: "), fullNameField),
New Paragraph(document, New Run(document, "Birth date: "), birthDateField),
New Paragraph(document, New Run(document, "Married: "), marriedField),
New Paragraph(document, New Run(document, "Gender: "), genderField)))
' Customize form fields.
Dim formFieldsData = document.Content.FormFieldsData
Dim fullNameFieldData = DirectCast(formFieldsData("FullName"), FormTextData)
fullNameFieldData.MaximumLength = 50
fullNameFieldData.ValueFormat = "Title case"
Dim birthdateFieldData = DirectCast(formFieldsData("BirthDate"), FormTextData)
birthdateFieldData.TextType = FormTextType.Date
birthdateFieldData.ValueFormat = "dd/MM/yyyy"
Dim marriedFieldData = DirectCast(formFieldsData("Married"), FormCheckBoxData)
' Status text is shown on status bar, a bottom-right corner of Microsoft Word.
marriedFieldData.StatusText = "Check if you're married."
' Help text is shown on field when in focus and F1 is pressed.
marriedFieldData.HelpText = "Check if you're married."
Dim genderFieldData = DirectCast(formFieldsData("Gender"), FormDropDownData)
' First item as a default option for non-selected value.
genderFieldData.Items.Add("<Select Gender>")
genderFieldData.Items.Add("Male")
genderFieldData.Items.Add("Female")
' Make document a form by restricting editing to filling-in form fields.
document.Protection.StartEnforcingProtection(EditingRestrictionType.FillingForms, "pass")
document.Save("Create Form.%OutputFileType%")
End Sub
End Module
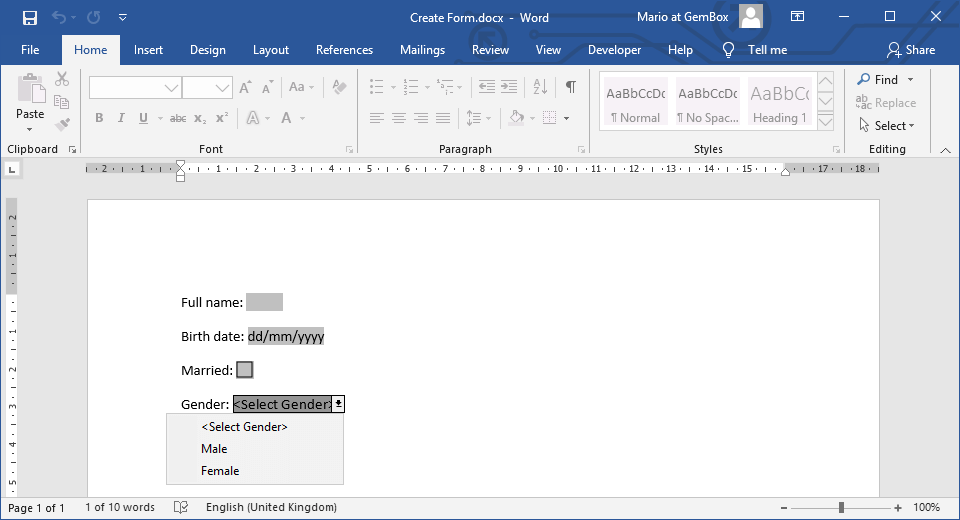
There are three types of form fields, each with an associated type of form data.
The Field.FieldType values: | The Field.FormData values: |
---|---|
FormText | FormTextData |
FormCheckBox | FormCheckBoxData |
FormDropDown | FormDropDownData |
To read more about Word document's protection and restriction, check out the Restrict Editing example.