Read Word Form Controls in C# and VB.NET
After you create a form and your user fills it, you'll want to read the filled-in values. GemBox.Document enables you to read the form field values of the whole document or just a part of a document by using ContentRange.FormFieldsData
collection.
For convenience, GemBox.Document provides form values as objects of different types. The value type depends on the type of the field and, in the case of a FormText
field, on the value of FormTextData.TextType
. You can read more about this on the FormFieldData.Value
remarks.
The following example shows how you can read text, check-box and drop-down form fields data.
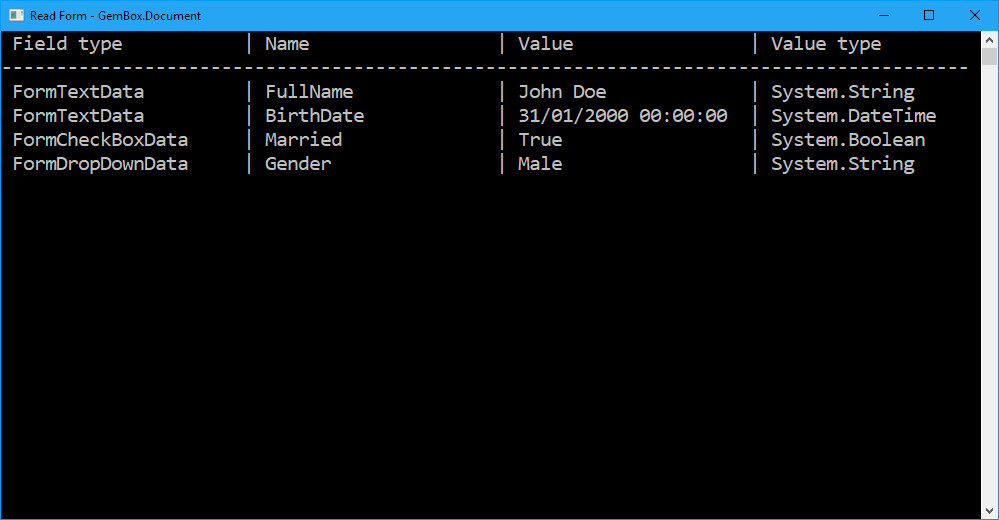
using GemBox.Document;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
// Get a snapshot of all form fields in the document.
FormFieldDataCollection formFieldsData = document.Content.FormFieldsData;
Console.WriteLine($" {"Field type",-20} | {"Name",-20} | {"Value",-20} | {"Value type",-20} ");
Console.WriteLine(new string('-', 88));
// Read type, name, value and value type of each form field in the document.
foreach (FormFieldData formFieldData in formFieldsData)
{
Type fieldType = formFieldData.GetType();
string fieldName = formFieldData.Name;
object fieldValue = formFieldData.Value;
Type valueType = fieldValue.GetType();
Console.WriteLine($" {fieldType.Name,-20} | {fieldName,-20} | {fieldValue,-20} | {valueType.FullName,-20} ");
}
}
}
Imports GemBox.Document
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%InputFileName%")
' Get a snapshot of all form fields in the document.
Dim formFieldsData As FormFieldDataCollection = document.Content.FormFieldsData
Console.WriteLine($" {"Field type",-20} | {"Name",-20} | {"Value",-20} | {"Value type",-20} ")
Console.WriteLine(New String("-"c, 88))
' Read type, name, value and value type of each form field in the document.
For Each formFieldData As FormFieldData In formFieldsData
Dim fieldType As Type = formFieldData.GetType()
Dim fieldName As String = formFieldData.Name
Dim fieldValue As Object = formFieldData.Value
Dim valueType As Type = fieldValue.GetType()
Console.WriteLine($" {fieldType.Name,-20} | {fieldName,-20} | {fieldValue,-20} | {valueType.FullName,-20} ")
Next
End Sub
End Module