Update Word Form Controls
The following example shows how you can write or modify values of an existing text, check-box, and drop-down form fields in a Word document using the GemBox.Document component.
using GemBox.Document;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%#FormFilled.docx%");
// Get a snapshot of all form fields in the document.
var formData = document.Content.FormFieldsData;
// Update "FullName" text field.
var fullNameData = (FormTextData)formData["FullName"];
fullNameData.Value = "Jane Doe";
// Update "BirthDate" text field.
var birthDateData = (FormTextData)formData["BirthDate"];
birthDateData.Value = new DateTime(2000, 2, 29);
// Check "Married" check-box field.
var marriedData = (FormCheckBoxData)formData["Married"];
marriedData.Value = true;
// Select "Female" from drop-down field.
var genderData = (FormDropDownData)formData["Gender"];
genderData.SelectedItemIndex = genderData.Items.IndexOf("Female");
document.Save("Form Updated.%OutputFileType%");
}
}
Imports GemBox.Document
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%#FormFilled.docx%")
' Get a snapshot of all form fields in the document.
Dim formData = document.Content.FormFieldsData
' Update "FullName" text field.
Dim fullNameData = DirectCast(formData("FullName"), FormTextData)
fullNameData.Value = "Jane Doe"
' Update "BirthDate" text field.
Dim birthDateData = DirectCast(formData("BirthDate"), FormTextData)
birthDateData.Value = New DateTime(2000, 2, 29)
' Check "Married" check-box field.
Dim marriedData = DirectCast(formData("Married"), FormCheckBoxData)
marriedData.Value = True
' Select "Female" from drop-down field.
Dim genderData = DirectCast(formData("Gender"), FormDropDownData)
genderData.SelectedItemIndex = genderData.Items.IndexOf("Female")
document.Save("Form Updated.%OutputFileType%")
End Sub
End Module
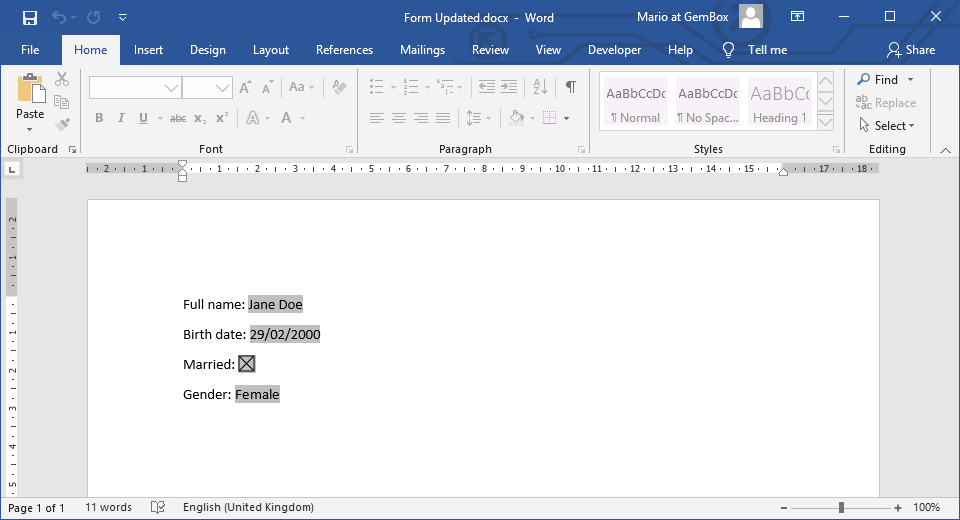
When setting the FormText
field's value to an IFormattable
type (like a number or date), the field's resulting text will depend on the value of FormTextData.ValueFormat
.