Unit Conversion
The following example shows how you can convert length units using the GemBox.Document component.
using GemBox.Document;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var document = DocumentModel.Load("%InputFileName%");
var pageSetup = document.Sections[0].PageSetup;
double widthInPoints = pageSetup.PageWidth;
double heightInPoints = pageSetup.PageHeight;
Console.WriteLine("Document's page size in different units:");
foreach (LengthUnit unit in Enum.GetValues(typeof(LengthUnit)))
{
double convertedWidth = LengthUnitConverter.Convert(widthInPoints, LengthUnit.Point, unit);
double convertedHeight = LengthUnitConverter.Convert(heightInPoints, LengthUnit.Point, unit);
Console.WriteLine($"{convertedWidth} x {convertedHeight} {unit.ToString().ToLowerInvariant()}");
}
}
}
Imports GemBox.Document
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim document = DocumentModel.Load("%InputFileName%")
Dim pageSetup = document.Sections(0).PageSetup
Dim widthInPoints As Double = pageSetup.PageWidth
Dim heightInPoints As Double = pageSetup.PageHeight
Console.WriteLine("Document's page size in different units:")
For Each unit As LengthUnit In [Enum].GetValues(GetType(LengthUnit))
Dim convertedWidth As Double = LengthUnitConverter.Convert(widthInPoints, LengthUnit.Point, unit)
Dim convertedHeight As Double = LengthUnitConverter.Convert(heightInPoints, LengthUnit.Point, unit)
Console.WriteLine($"{convertedWidth} x {convertedHeight} {unit.ToString().ToLowerInvariant()}")
Next
End Sub
End Module
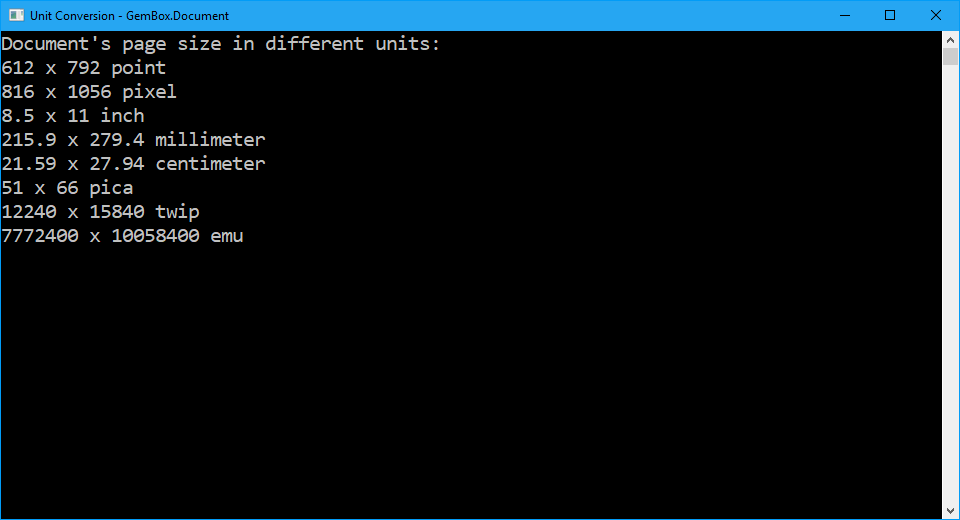
The LengthUnitConverter.Convert
method converts:
- 1 pixel to 0.75 points.
- 1 inch to 72 points.
- 1 millimeter to ~2.83 points.
- 1 centimeter to ~28.3 points.
- 1 pica to 12 points.
- 1 twip to 0.05 points.
- 1 emu to ~7.87e-5 points.