Export Word to XpsDocument in WPF
Besides converting a document to a different file format (like PDF, HTML, and image), GemBox.Document also supports converting a document to an XpsDocument
object with the DocumentModel.ConvertToXpsDocument
method.
If you require more than displaying a file's content, for instance if you want to be able to modify it with some Graphical User Interface (GUI) control, take a look at this Word Editor in WPF example.
The following example shows how you can convert a Word file to XPS and attach it to WPF's DocumentViewer
control.
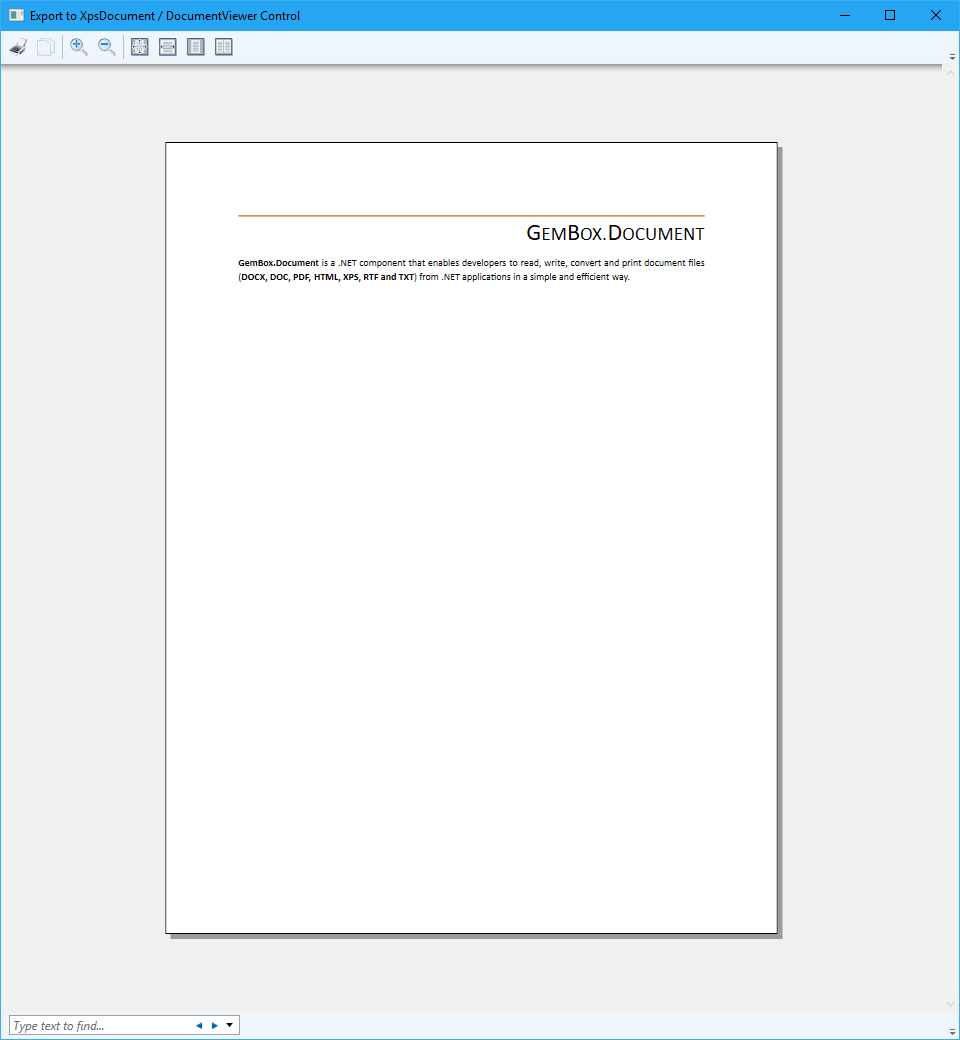
<Window x:Class="ExportToXpsDocument.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Export to XpsDocument / DocumentViewer Control"
SizeToContent="WidthAndHeight">
<DocumentViewer x:Name="DocumentViewer"/>
</Window>
using GemBox.Document;
using System.Windows;
using System.Windows.Xps.Packaging; // Add reference for 'ReachFramework'.
namespace ExportToXpsDocument
{
public partial class MainWindow : Window
{
private XpsDocument xpsDocument;
public MainWindow()
{
this.InitializeComponent();
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
this.SetDocumentViewer("%#Reading.docx%");
}
private void SetDocumentViewer(string path)
{
var document = DocumentModel.Load(path);
// XpsDocument object must stay referenced so that DocumentViewer can access its resources.
// Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will no longer work.
this.xpsDocument = document.ConvertToXpsDocument(SaveOptions.XpsDefault);
this.DocumentViewer.Document = this.xpsDocument.GetFixedDocumentSequence();
}
}
}
Imports GemBox.Document
Imports System.Windows
Imports System.Windows.Xps.Packaging ' Add reference for 'ReachFramework'.
Namespace ExportToXpsDocument
Partial Public Class MainWindow
Inherits Window
Private xpsDocument As XpsDocument
Public Sub New()
Me.InitializeComponent()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Me.SetDocumentViewer("%#Reading.docx%")
End Sub
Private Sub SetDocumentViewer(path As String)
Dim document = DocumentModel.Load(path)
' XpsDocument object must stay referenced so that DocumentViewer can access its resources.
' Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will no longer work.
Me.xpsDocument = document.ConvertToXpsDocument(SaveOptions.XpsDefault)
Me.DocumentViewer.Document = Me.xpsDocument.GetFixedDocumentSequence()
End Sub
End Class
End Namespace