Email message flags
The example demonstrates how to add, list, and remove an IMAP flag using the GemBox.Email library in C# and VB.NET.
using GemBox.Email;
using GemBox.Email.Imap;
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (ImapClient imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
// Select INBOX folder.
imap.SelectInbox();
// Add "Draft" flag to first message.
imap.AddMessageFlags(1, ImapMessageFlags.Draft);
// Get first message flags and display them.
// Notice the presence of "Draft" flag.
IList<string> flags = imap.GetMessageFlags(1);
foreach (string flag in flags)
Console.WriteLine(flag);
// Remove "Draft" flag from first message.
imap.RemoveMessageFlags(1, ImapMessageFlags.Draft);
Console.WriteLine(new string('-', 10));
// Again, get first message flags and display them.
// Notice the absence of "Draft" flag.
flags = imap.GetMessageFlags(1);
foreach (string flag in flags)
Console.WriteLine(flag);
}
}
}
Imports GemBox.Email
Imports GemBox.Email.Imap
Imports System
Imports System.Collections.Generic
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using imap As New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
' Select INBOX folder.
imap.SelectInbox()
' Add "Draft" flag to first message.
imap.AddMessageFlags(1, ImapMessageFlags.Draft)
' Get first message flags and display them.
' Notice the presence of "Draft" flag.
Dim flags As IList(Of String) = imap.GetMessageFlags(1)
For Each flag As String In flags
Console.WriteLine(flag)
Next
' Remove "Draft" flag from first message.
imap.RemoveMessageFlags(1, ImapMessageFlags.Draft)
Console.WriteLine(New String("-"c, 10))
' Again, get first message flags and display them.
' Notice the absence of "Draft" flag.
flags = imap.GetMessageFlags(1)
For Each flag As String In flags
Console.WriteLine(flag)
Next
End Using
End Sub
End Module
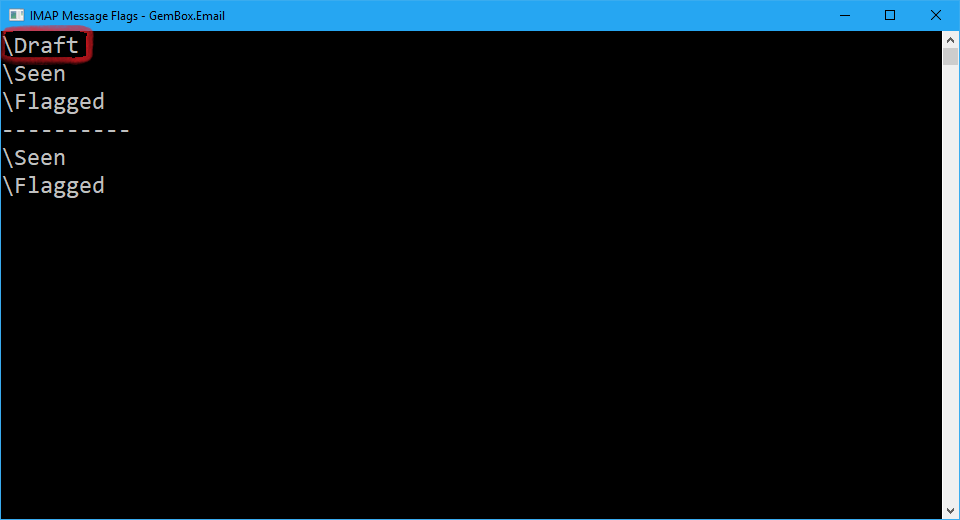
Besides working with just a single message's flags (such as ImapClient.AddMessageFlags
methods), you can also process the flags for a group of messages with methods that accept the message range, by defining the start and end message number (such as ImapClient.AddMessageRangeFlags
methods).
For more information about ImapClient
, check out our IMAP Client Connection example.