List and modify folders on Microsoft 365 Server
The example below shows how you can list all folders on Microsoft 365 (Office 365) server, create a new one, and then remove it using the GemBox.Email library in C# and VB.NET.
using GemBox.Email;
using GemBox.Email.Graph;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Create a new Graph client.
var graphClient = new GraphClient();
graphClient.Authenticate("<OAUTH2.0-TOKEN>");
graphClient.ImpersonateUser("<USER-ID-OR-EMAIL>");
// Create a new folder.
graphClient.CreateFolder("GemBox Folder");
// List folders on the server.
var folders = graphClient.ListFolders();
// Print folder info.
Console.WriteLine("Folder name".PadRight(28, ' ') + " | Items | Unread items | Children folders");
foreach (var folder in folders)
Console.WriteLine(
$"{folder.Name,-28} | " +
$"{folder.TotalCount,-5} | " +
$"{folder.UnreadCount,-12} | " +
$"{folder.ChildFolderCount,-16}");
// Delete a folder.
graphClient.DeleteFolder("GemBox Folder");
}
}
Imports GemBox.Email
Imports GemBox.Email.Graph
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Create a new Graph client.
Dim graphClient = New GraphClient()
graphClient.Authenticate("<OAUTH2.0-TOKEN>")
graphClient.ImpersonateUser("<USER-ID-OR-EMAIL>")
' Create a new folder.
graphClient.CreateFolder("GemBox Folder")
' List folders on the server.
Dim folders = graphClient.ListFolders()
' Print folder info.
Console.WriteLine("Folder name".PadRight(28, " "c) & " | Items | Unread items | Children folders")
For Each folder In folders
Console.WriteLine(
$"{folder.Name,-28} | " +
$"{folder.TotalCount,-5} | " +
$"{folder.UnreadCount,-12} | " +
$"{folder.ChildFolderCount,-16}")
Next
' Delete a folder.
graphClient.DeleteFolder("GemBox Folder")
End Sub
End Module
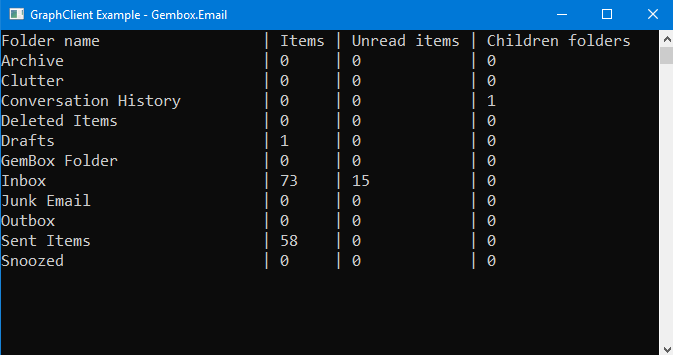
To modify folders on the Office 365 server, you can use the GraphClient.CreateFolder
, GraphClient.DeleteFolder
, and GraphClient.RenameFolder
methods. With the GraphClient.GetFolderInfo
method, you can obtain information about folders like name, total messages count and unread messages count.