Upload an Email Message with IMAP
The following example shows how you can upload an email message to the INBOX folder via IMAP protocol using GemBox.Email in your C# and VB.NET applications.
using GemBox.Email;
using GemBox.Email.Imap;
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (ImapClient imap = new ImapClient("<ADDRESS> (e.g. imap.gmail.com)"))
{
imap.Connect();
imap.Authenticate("<USERNAME>", "<PASSWORD>");
// Create new email message.
MailMessage message = new MailMessage(
new MailAddress("sender@example.com", "Sender"),
new MailAddress("first.receiver@example.com", "First receiver"),
new MailAddress("second.receiver@example.com", "Second receiver"));
// Add additional receivers.
message.Cc.Add(
new MailAddress("third.receiver@example.com", "Third receiver"),
new MailAddress("fourth.receiver@example.com", "Fourth receiver"));
// Add subject and body.
message.Subject = "Upload Email in C# / VB.NET / ASP.NET";
message.BodyText = "Hi 👋,\n" +
"This message was created and uploaded with GemBox.Email.\n" +
"Read more about it on https://www.gemboxsoftware.com/email";
// Uploads the message to the INBOX folder
imap.UploadMessage(message, "INBOX");
}
}
}
Imports GemBox.Email
Imports GemBox.Email.Imap
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using imap As ImapClient = New ImapClient("<ADDRESS> (e.g. imap.gmail.com)")
imap.Connect()
imap.Authenticate("<USERNAME>", "<PASSWORD>")
' Create new email message.
Dim message As New MailMessage(
New MailAddress("sender@example.com", "Sender"),
New MailAddress("first.receiver@example.com", "First receiver"),
New MailAddress("second.receiver@example.com", "Second receiver"))
' Add additional receivers.
message.Cc.Add(
New MailAddress("third.receiver@example.com", "Third receiver"),
New MailAddress("fourth.receiver@example.com", "Fourth receiver"))
' Add subject and body.
message.Subject = "Upload Email in C# / VB.NET / ASP.NET"
message.BodyText = "Hi 👋," & vbLf &
"This message was created and uploaded with GemBox.Email." & vbLf &
"Read more about it on https://www.gemboxsoftware.com/email"
' Uploads the message to the INBOX folder
imap.UploadMessage(message, "INBOX")
End Using
End Sub
End Module
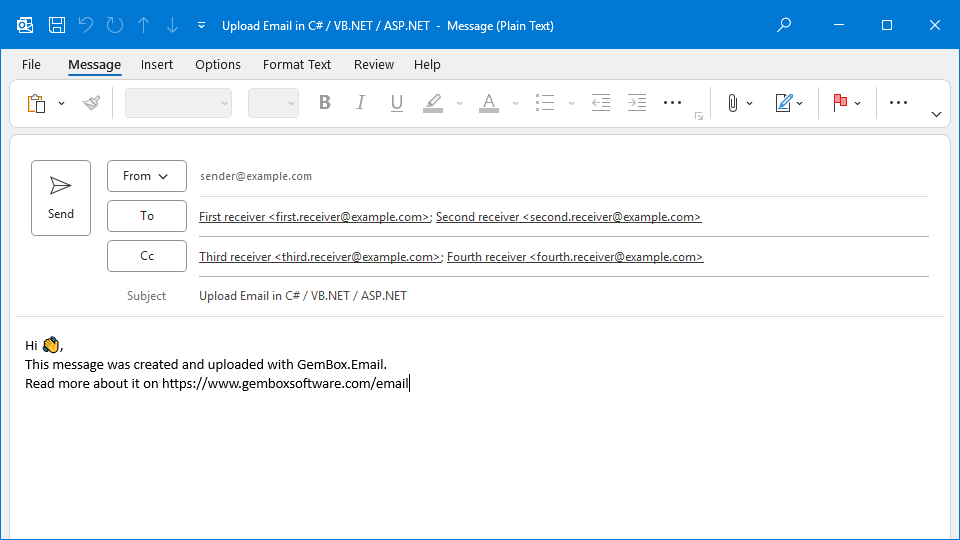
You can use the ImapClient.UploadMessage
method to save the email message in the desired folder. Note that this method will not send the email message to its recipients. If you are looking for that, check out our sending example.