Apply filters to images
With GemBox.Imaging, you can apply filters to images programmatically in C# and VB.NET, as shown in the following example.
using GemBox.Imaging;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var image = Image.Load("%InputFileName%"))
{
// Adjust blue channel and contrast of the image.
image.ApplyFilter(x => x
.Blue(50)
.Contrast(20)
);
// Save the adjusted image.
image.Save("Adjusted.png");
}
}
}
Imports GemBox.Imaging
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using image As Image = Image.Load("%InputFileName%")
' Adjust blue channel and contrast of the image.
image.ApplyFilter(
Function(x) x _
.Blue(50) _
.Contrast(20)
)
' Save the adjusted image.
image.Save("Adjusted.png")
End Using
End Sub
End Module
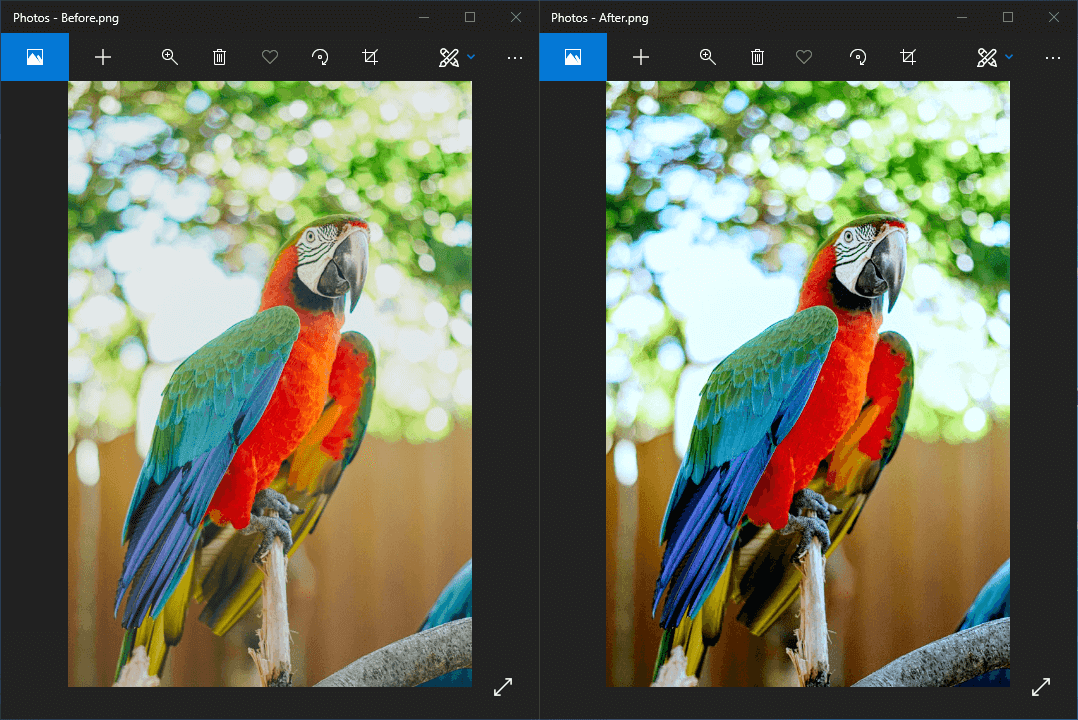
To apply filters to an image using GemBox.Imaging you need to call the Image.ApplyFilter(ColorFilterBuilder)
method and specify the filters you want to apply by calling methods of the FilterBuilder
:
Red
: Sets the red channel intensity.Green
: Sets the green channel intensity.Blue
: Sets the blue channel intensity.Alpha
: Sets the alpha channel intensity (transparency).Brightness
: Sets brightness.Contrast
: Sets contrast.Hue
: Sets hueSaturation
: Sets saturation (chroma).