Resize image files
The following example demonstrates how to resize an image and save it to PNG format in C# and VB.NET using GemBox.Imaging on multiple platforms.
using GemBox.Imaging;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var image = Image.Load("%InputFileName%"))
{
// Resize the image to 128 x 85 pixels.
image.Resize(128, 85);
// Save the resized image.
image.Save("Resized.jpg");
}
}
}
Imports GemBox.Imaging
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using image As Image = Image.Load("%InputFileName%")
' Resize the image to 128 x 85 pixels.
image.Resize(128, 85)
' Save the resized image.
image.Save("Resized.jpg")
End Using
End Sub
End Module
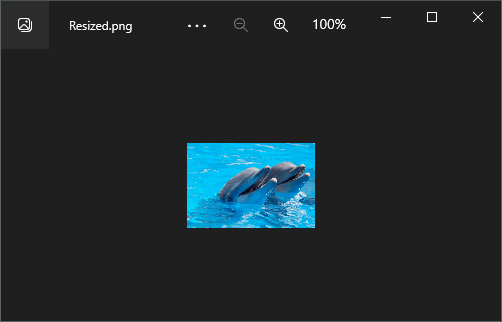
If you want to keep the original aspect ratio, provide just one value. E.g. you can use Image.Resize(128, null) to resize the image to a width of 128 pixels while the height value will be automatically calculated.
Resize a TIFF image frame
With GemBox.Imaging, you can resize individual frames of an image programmatically, as shown in the following example.
using GemBox.Imaging;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var image = Image.Load("%#parrots.tiff%"))
{
// Get the middle frame and reduce its size by half.
var frame = image.Frames[image.Frames.Count / 2];
frame.Resize(frame.Width / 2, frame.Height / 2);
// Save the resized image.
image.Save("Resized.tiff");
}
}
}
Imports GemBox.Imaging
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using image = GemBox.Imaging.Image.Load("%#parrots.tiff%")
' Get the middle frame and reduce its size by half.
Dim frame = image.Frames(image.Frames.Count \ 2)
frame.Resize(frame.Width \ 2, frame.Height \ 2)
' Save the resized image.
image.Save("Resized.tiff")
End Using
End Sub
End Module
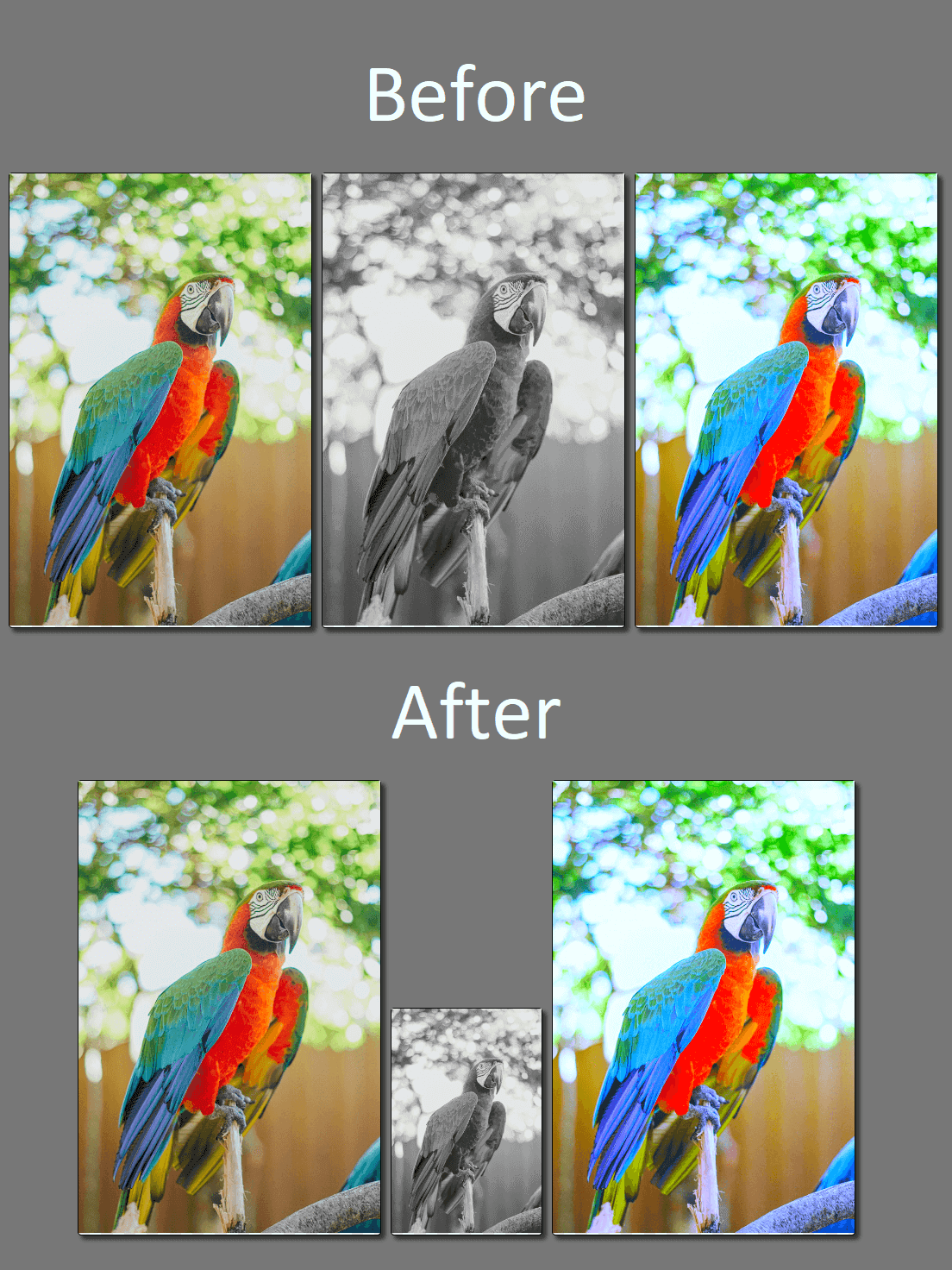