Flatten PDF interactive form fields
The following example shows how to flatten the PDF interactive form with GemBox.Pdf.
using GemBox.Pdf;
using GemBox.Pdf.Content;
using GemBox.Pdf.Forms;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
using (var document = PdfDocument.Load("%InputFileName%"))
{
// A flag specifying whether to construct appearance for all form fields in the document.
bool needAppearances = document.Form.NeedAppearances;
foreach (var field in document.Form.Fields)
{
// Do not flatten button fields.
if (field.FieldType == PdfFieldType.Button)
continue;
// Construct appearance, if needed.
if (needAppearances)
field.Appearance.Refresh();
// Get the field's appearance form.
var fieldAppearance = field.Appearance.Get();
// If the field doesn't have an appearance, skip it.
if (fieldAppearance == null)
continue;
// Draw field's appearance on the page.
field.Page.Content.DrawAnnotation(field);
}
// Remove all fields, thus making the document non-interactive,
// since their appearance is now contained directly in the content of their pages.
document.Form.Fields.Clear();
document.Save("FormFlattened.%OutputFileType%");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Content
Imports GemBox.Pdf.Forms
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Using document = PdfDocument.Load("%InputFileName%")
' A flag specifying whether to construct appearance for all form fields in the document.
Dim needAppearances = document.Form.NeedAppearances
For Each field In document.Form.Fields
' Do not flatten button fields.
If field.FieldType = PdfFieldType.Button Then Continue For
' Construct appearance, if needed.
if needAppearances Then field.Appearance.Refresh()
' Get the field's appearance form.
Dim fieldAppearance = field.Appearance.Get()
' If the field doesn't have an appearance, skip it.
If fieldAppearance Is Nothing Then Continue For
' Draw field's appearance on the page.
field.Page.Content.DrawAnnotation(field)
Next
' Remove all fields, thus making the document non-interactive,
' since their appearance is now contained directly in the content of their pages.
document.Form.Fields.Clear()
document.Save("FormFlattened.%OutputFileType%")
End Using
End Sub
End Module
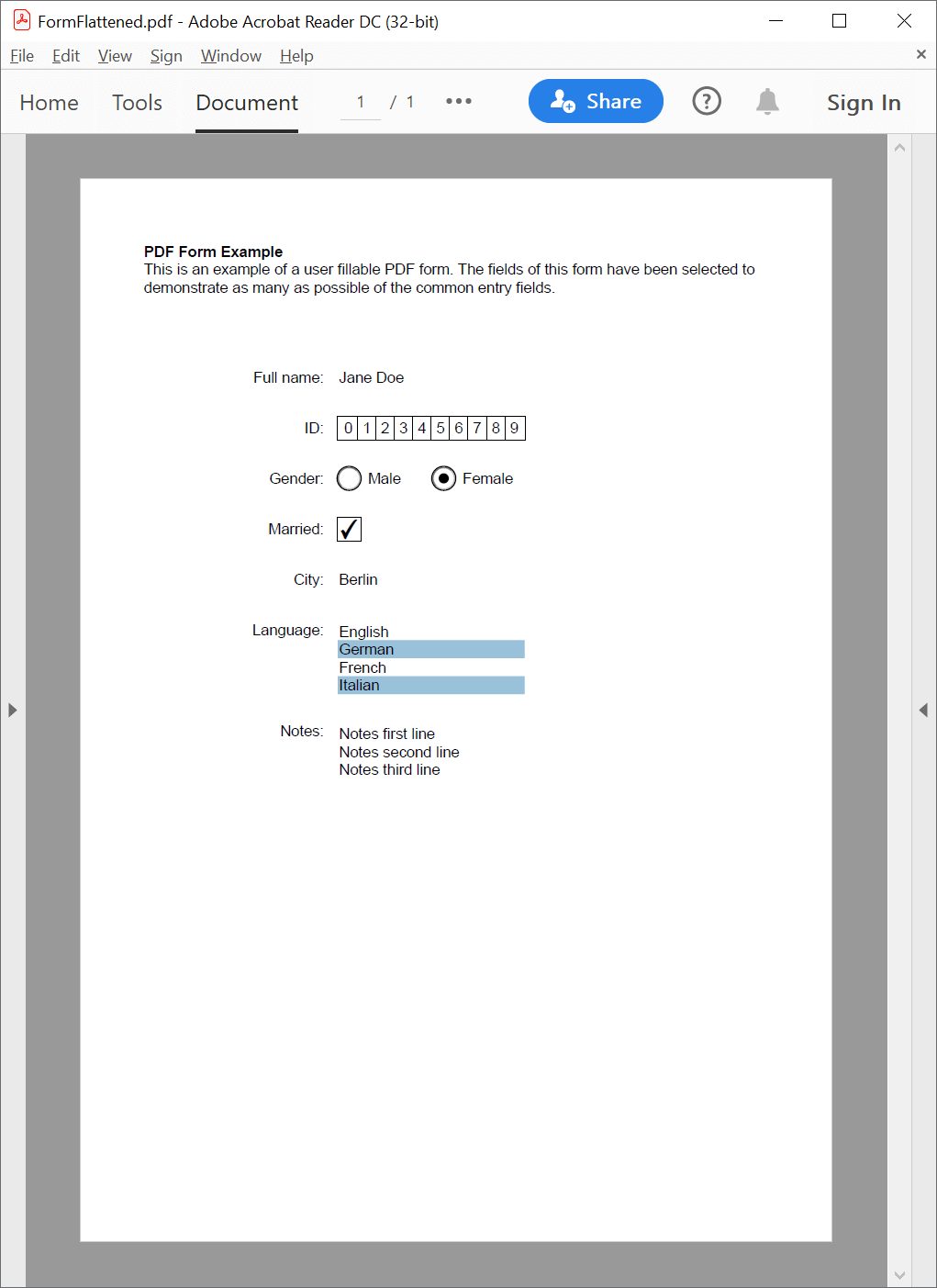
Using the same approach, you can also flatten PdfAnnotation
s contained in the Annotations property of the PdfPage
class.
Another approach of making the PDF form non-interactive is to make all form fields read-only using the ReadOnly property of the PdfField
class.