Decrypt and Encrypt PDF files
With the examples below, you will be able to understand how to use GemBox.Pdf for PDF file encryption with for each of the following scenarios:
- Encrypt a PDF file
- Restrict editing of a PDF file
- Specify encryption settings
- Decrypt a PDF file
- Retrying decryption
Encrypt a PDF file
See the example below to learn how to encrypt an existing PDF file with a password.
using GemBox.Pdf;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load PDF document from an unencrypted PDF file.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Set password-based encryption with password required to open a PDF document.
document.SaveOptions.SetPasswordEncryption().DocumentOpenPassword = "%DocumentOpenPassword%";
// Save PDF document to an encrypted PDF file.
document.Save("Encryption.pdf");
}
}
}
Imports GemBox.Pdf
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load PDF document from an unencrypted PDF file.
Using document = PdfDocument.Load("%InputFileName%")
' Set password-based encryption with password required to open a PDF document.
document.SaveOptions.SetPasswordEncryption().DocumentOpenPassword = "%DocumentOpenPassword%"
' Save PDF document to an encrypted PDF file.
document.Save("Encryption.pdf")
End Using
End Sub
End Module
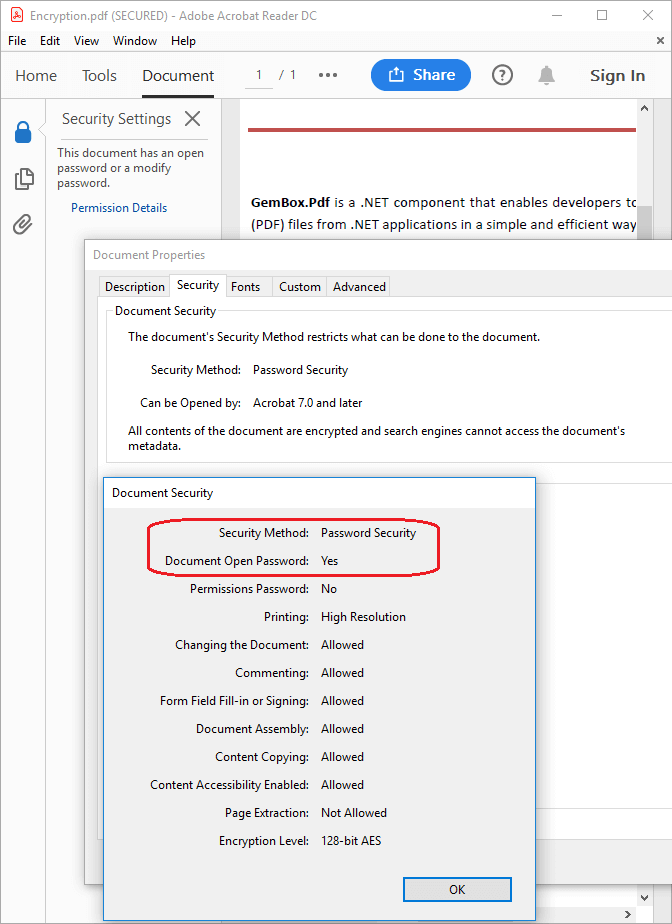
As shown in the screenshot from above, the output PDF file has a Password Security method, and Document Open Password is required to open a PDF file.
You can perform all operations except page extraction. The Restrict Editing example shows how to modify user access permissions.
The PDF encryption level is 128-bit AES and is supported in Adobe Acrobat 7.0 and later. All contents of the document are encrypted, including metadata (used by search engines). The Encryption Settings example shows how to modify the encryption level and options.
Restrict editing of a PDF file
If you need to restrict users from editing PDF files, see the example below.
using GemBox.Pdf;
using GemBox.Pdf.Security;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load PDF document from an unencrypted PDF file.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Set password-based encryption to an output PDF file.
var encryption = document.SaveOptions.SetPasswordEncryption();
// Specify the password required to edit a PDF document.
encryption.PermissionsPassword = "%PermissionsPassword%";
// User will be able to print PDF and fill in the PDF form
// without requiring a password.
encryption.Permissions =
PdfUserAccessPermissions.Print |
PdfUserAccessPermissions.FillForm |
PdfUserAccessPermissions.CopyContentForAccessibility |
PdfUserAccessPermissions.PrintHighResolution;
// Save the PDF document to an encrypted PDF file.
document.Save("Restrict Editing.pdf");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Security
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load PDF document from an unencrypted PDF file.
Using document = PdfDocument.Load("%InputFileName%")
' Set password-based encryption to an output PDF file.
Dim encryption = document.SaveOptions.SetPasswordEncryption()
' Specify the password required to edit a PDF document.
encryption.PermissionsPassword = "%PermissionsPassword%"
' User will be able to print PDF and fill in the PDF form
' without requiring a password.
encryption.Permissions =
PdfUserAccessPermissions.Print Or
PdfUserAccessPermissions.FillForm Or
PdfUserAccessPermissions.CopyContentForAccessibility Or
PdfUserAccessPermissions.PrintHighResolution
' Save the PDF document to an encrypted PDF file.
document.Save("Restrict Editing.pdf")
End Using
End Sub
End Module
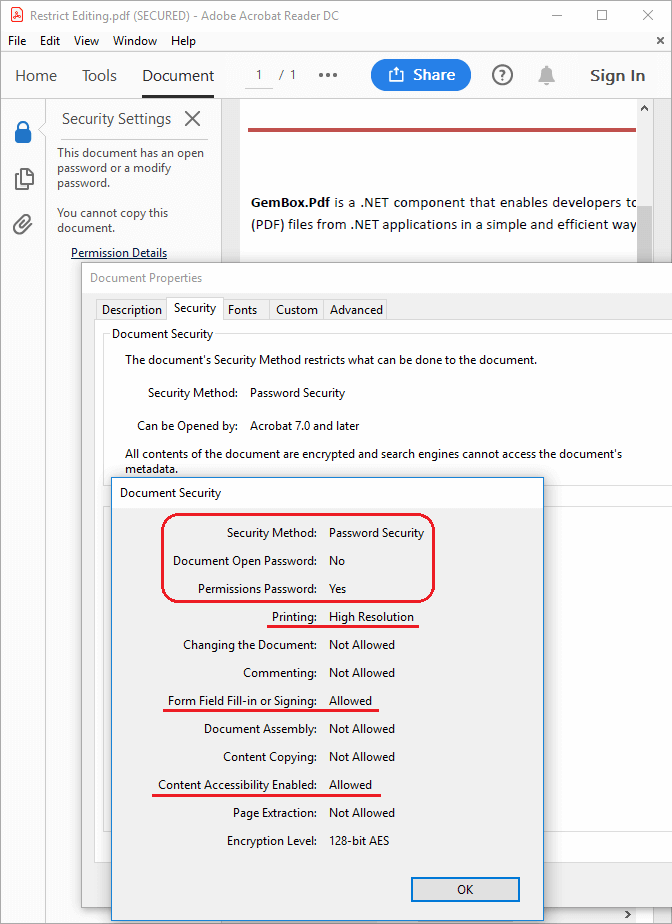
Note that the PDF viewer/editor does the enforcement of user access permissions. You can open and bypass those restrictions in a PDF file by using applications that do not respect PDF specification requirements.
GemBox.Pdf does not respect PDF specification requirements regarding user access permissions because it is not an end-user application. Also keep in mind that although a PDF file is encrypted, a password is not necessary to open it.
Specify encryption settings
The following example shows how to encrypt an existing PDF file using various encryption settings.
using GemBox.Pdf;
using GemBox.Pdf.Security;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// Load PDF document from an unencrypted PDF file.
using (var document = PdfDocument.Load("%InputFileName%"))
{
// Set password-based encryption to an output PDF file.
var encryption = document.SaveOptions.SetPasswordEncryption();
// Specify password required to open a PDF document.
encryption.DocumentOpenPassword = "%DocumentOpenPassword%";
// Specify password required to edit a PDF document.
encryption.PermissionsPassword = "%PermissionsPassword%";
// User will be able to print PDF and fill-in PDF form
// without requiring a password.
encryption.Permissions =
PdfUserAccessPermissions.Print |
PdfUserAccessPermissions.FillForm |
PdfUserAccessPermissions.CopyContentForAccessibility |
PdfUserAccessPermissions.PrintHighResolution;
// Specify 256-bit AES encryption level (supported in Acrobat X and later).
encryption.EncryptionLevel = new PdfEncryptionLevel(PdfEncryptionAlgorithm.AES, 256);
// Encrypt content and embedded files but do not encrypt document's metadata.
encryption.Options = PdfEncryptionOptions.EncryptContent | PdfEncryptionOptions.EncryptEmbeddedFiles;
// Save PDF document to an encrypted PDF file.
document.Save("Encryption Settings.pdf");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Security
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' Load PDF document from an unencrypted PDF file.
Using document = PdfDocument.Load("%InputFileName%")
' Set password-based encryption to an output PDF file.
Dim encryption = document.SaveOptions.SetPasswordEncryption()
' Specify password required to open a PDF document.
encryption.DocumentOpenPassword = "%DocumentOpenPassword%"
' Specify password required to edit a PDF document.
encryption.PermissionsPassword = "%PermissionsPassword%"
' User will be able to print PDF and fill-in PDF form
' without requiring a password.
encryption.Permissions =
PdfUserAccessPermissions.Print Or
PdfUserAccessPermissions.FillForm Or
PdfUserAccessPermissions.CopyContentForAccessibility Or
PdfUserAccessPermissions.PrintHighResolution
' Specify 256-bit AES encryption level (supported in Acrobat X and later).
encryption.EncryptionLevel = New PdfEncryptionLevel(PdfEncryptionAlgorithm.AES, 256)
' Encrypt content and embedded files but do not encrypt document's metadata.
encryption.Options = PdfEncryptionOptions.EncryptContent Or PdfEncryptionOptions.EncryptEmbeddedFiles
' Save PDF document to an encrypted PDF file.
document.Save("Encryption Settings.pdf")
End Using
End Sub
End Module
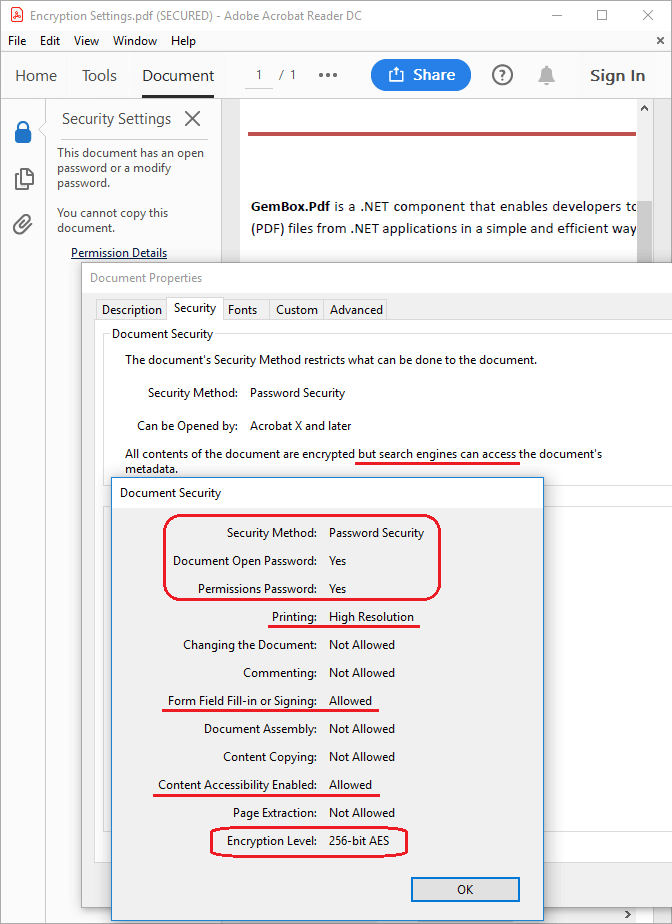
The first part of this example is a combination of Encrypt and Restrict Editing examples so a password (either the DocumentOpenPassword
or the PermissionsPassword
) will be required to open a document and only users who entered a PermissionsPassword
will be able to edit a document in a PDF editor application.
The last part of this example shows how to set the encryption to the strongest level currently supported by PDF password-based encryption – 256-bit AES supported by Adobe Acrobat X and later.
It also shows how to specify which parts of a PDF file should be encrypted. In this example, the document's metadata stream is excluded from encryption so search engines will be able to access it.
Decrypt a PDF file
The following example shows how to load an encrypted PDF file, remove the encryption, and then save it as an unencrypted PDF file.
using GemBox.Pdf;
using GemBox.Pdf.Security;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
try
{
// Load PDF document from a potentially encrypted PDF file.
using (var document = PdfDocument.Load("%InputFileName%",
new PdfLoadOptions() { Password = "%DocumentOpenPassword%" }))
{
// Remove encryption from an output PDF file.
document.SaveOptions.Encryption = null;
// Save PDF document to an unencrypted PDF file.
document.Save("Decryption.pdf");
}
}
catch (InvalidPdfPasswordException ex)
{
// Gracefully handle the case when input PDF file is encrypted
// and provided password is invalid.
Console.WriteLine(ex.Message);
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Security
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Try
' Load PDF document from a potentially encrypted PDF file.
Using document = PdfDocument.Load("%InputFileName%",
New PdfLoadOptions() With {.Password = "%DocumentOpenPassword%"})
' Remove encryption from an output PDF file.
document.SaveOptions.Encryption = Nothing
'Save PDF document to an unencrypted PDF file.
document.Save("Decryption.pdf")
End Using
Catch ex As InvalidPdfPasswordException
' Gracefully handle the case when input PDF file is encrypted
' and provided password is invalid.
Console.WriteLine(ex.Message)
End Try
End Sub
End Module
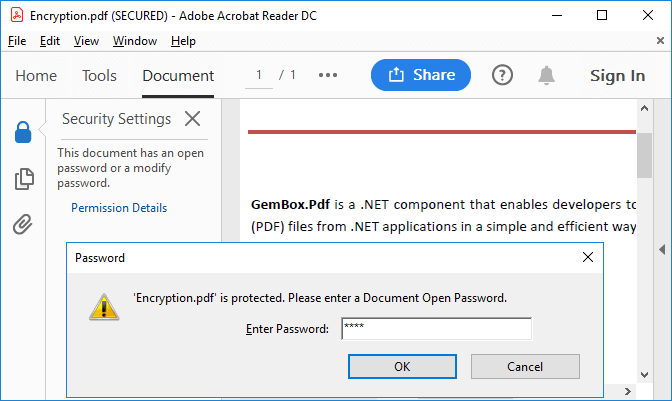
To decrypt a PDF file when loading a PDF document, either the DocumentOpenPassword
or the PermissionsPassword
must be specified in the PdfLoadOptions.Password
property. If the specified password is invalid, then an InvalidPdfPasswordException
is thrown.
If you just want to check if the PDF file is encrypted, then you can load the PDF file without providing a password and check if the PdfDocument.SaveOptions.Encryption
is not null
.
If the input PDF file is not encrypted or is encrypted but with an empty DocumentOpenPassword
(like in the Restrict Editing example), then the password specified in the PdfLoadOptions.Password
property is not used.
Note that GemBox.Pdf verifies the password only when the first PdfString
or PdfStream
from an encrypted PDF file has to be read, since only strings and streams are subject to encryption, based on the PDF specification. As a consequence, an InvalidPdfPasswordException
might be thrown after the loading of a PDF document.
This behavior enables you to extract some useful information from an encrypted PDF file without knowing its DocumentOpenPassword
password, like number of pages or other information that doesn't directly reference any PdfString
or PdfStream
.
Retrying decryption
The following example shows how you can try decrypting an encrypted PDF file with multiple passwords using the PdfLoadOptions.LoadingEncrypted
event. Note that the event only fires when reading encrypted files.
using GemBox.Pdf;
using GemBox.Pdf.Security;
using System;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var loadOptions = new PdfLoadOptions();
loadOptions.AuthorizationOnDocumentOpen = true;
loadOptions.LoadingEncrypted += (sender, e) =>
{
Console.WriteLine("PDF file is encrypted, please enter the password:");
bool wrongPassword;
do
{
string password = Console.ReadLine();
if (string.IsNullOrEmpty(password))
break;
wrongPassword = !e.SetPassword(password);
if (wrongPassword)
Console.WriteLine("The password is incorrect, please try again:");
}
while (wrongPassword);
};
try
{
using (var document = PdfDocument.Load("%#Encryption.pdf%", loadOptions))
{
Console.WriteLine("The correct password was provided.");
}
}
catch (InvalidPdfPasswordException)
{
Console.WriteLine("The incorrect password was provided.");
}
}
}
Imports GemBox.Pdf
Imports GemBox.Pdf.Security
Imports System
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim loadOptions As New PdfLoadOptions()
loadOptions.AuthorizationOnDocumentOpen = True
AddHandler loadOptions.LoadingEncrypted,
Sub(sender, e)
Console.WriteLine("PDF file is encrypted, please enter the password:")
Dim wrongPassword As Boolean
Do
Dim password As String = Console.ReadLine()
If String.IsNullOrEmpty(password) Then Exit Do
wrongPassword = Not e.SetPassword(password)
If wrongPassword Then Console.WriteLine("The password is incorrect, please try again:")
Loop While wrongPassword
End Sub
Try
Using document = PdfDocument.Load("%#Encryption.pdf%", LoadOptions)
Console.WriteLine("The correct password was provided.")
End Using
Catch ex As InvalidPdfPasswordException
Console.WriteLine("The incorrect password was provided.")
End Try
End Sub
End Module
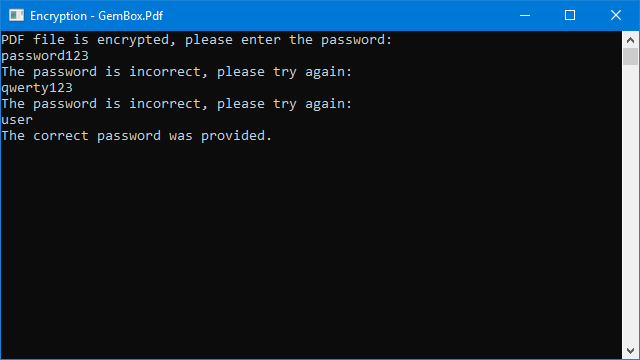