Export PDF in WPF
The following example shows how to convert a PDF file to an XpsDocument
instance and attach it to WPF's DocumentViewer
control.
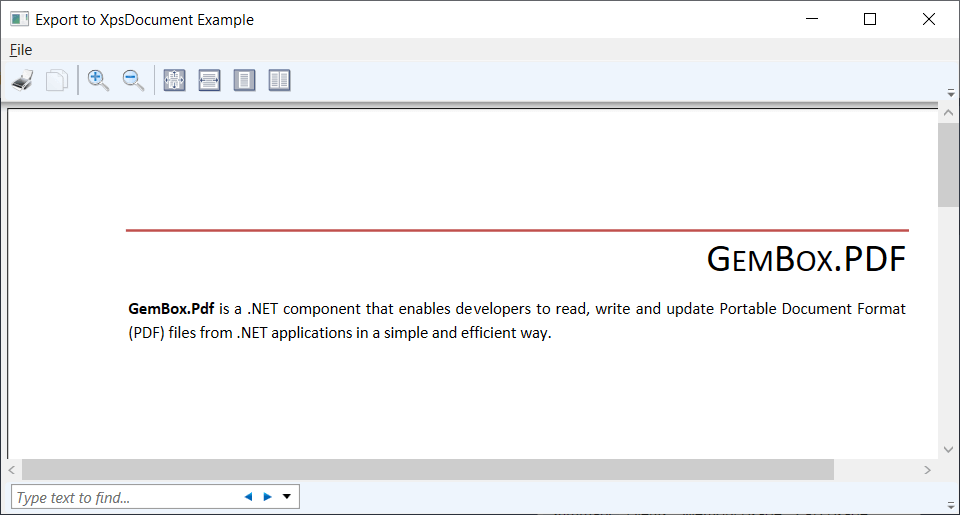
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Export to XpsDocument Example"
SizeToContent="WidthAndHeight">
<DockPanel>
<Menu DockPanel.Dock="Top">
<MenuItem Header="_File">
<MenuItem Header="_Open" Click="MenuItem_Click" />
</MenuItem>
</Menu>
<DocumentViewer x:Name="DocumentViewer"/>
</DockPanel>
</Window>
using GemBox.Pdf;
using Microsoft.Win32;
using System.Windows;
using System.Windows.Xps.Packaging;
public partial class MainWindow : Window
{
XpsDocument xpsDocument;
public MainWindow()
{
InitializeComponent();
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
private void MenuItem_Click(object sender, RoutedEventArgs e)
{
OpenFileDialog fileDialog = new OpenFileDialog();
fileDialog.Filter = "PDF files (*.pdf)|*.pdf";
if (fileDialog.ShowDialog() == true)
{
using (var document = PdfDocument.Load(fileDialog.FileName))
{
// XpsDocument needs to stay referenced so that DocumentViewer can access additional required resources.
// Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will not work.
this.xpsDocument = document.ConvertToXpsDocument(SaveOptions.Xps);
this.DocumentViewer.Document = this.xpsDocument.GetFixedDocumentSequence();
}
}
}
}
Imports GemBox.Pdf
Imports Microsoft.Win32
Imports System.Windows.Xps.Packaging
Class MainWindow
Dim xpsDoc As XpsDocument
Public Sub New()
InitializeComponent()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
End Sub
Private Sub MenuItem_Click(sender As Object, e As RoutedEventArgs)
Dim fileDialog = New OpenFileDialog()
fileDialog.Filter = "PDF files (*.pdf)|*.pdf"
If (fileDialog.ShowDialog() = True) Then
Using document = PdfDocument.Load(fileDialog.FileName)
' XpsDocument needs to stay referenced so that DocumentViewer can access additional required resources.
' Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will not work.
Me.xpsDoc = document.ConvertToXpsDocument(SaveOptions.Xps)
Me.DocumentViewer.Document = Me.xpsDoc.GetFixedDocumentSequence()
End Using
End If
End Sub
End Class
Export PDF to ImageSource in WPF
The following example shows how to convert a PDF page to an ImageSource instance and attach it to WPF's Image control, using GemBox.Pdf.
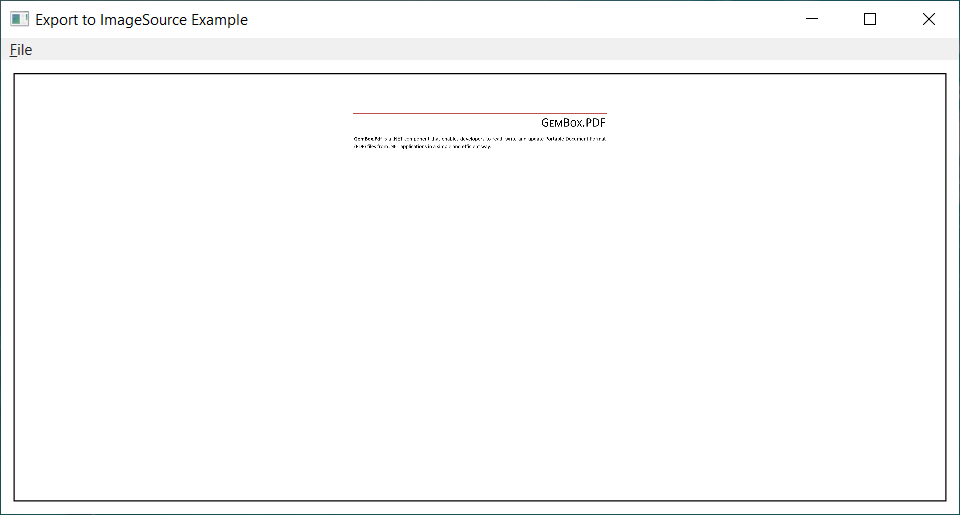
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Export to ImageSource Example"
SizeToContent="WidthAndHeight">
<DockPanel>
<Menu DockPanel.Dock="Top">
<MenuItem Header="_File">
<MenuItem Header="_Open" Click="MenuItem_Click" />
</MenuItem>
</Menu>
<Border Margin="10" BorderBrush="Black" BorderThickness="1">
<Image x:Name="ImageControl"/>
</Border>
</DockPanel>
</Window>
using GemBox.Pdf;
using Microsoft.Win32;
using System.Windows;
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
}
private void MenuItem_Click(object sender, RoutedEventArgs e)
{
OpenFileDialog fileDialog = new OpenFileDialog();
fileDialog.Filter = "PDF files (*.pdf)|*.pdf";
if (fileDialog.ShowDialog() == true)
{
using (var document = PdfDocument.Load(fileDialog.FileName))
{
this.ImageControl.Source = document.ConvertToImageSource(SaveOptions.Image);
}
}
}
}
Imports GemBox.Pdf
Imports Microsoft.Win32
Class MainWindow
Public Sub New()
InitializeComponent()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
End Sub
Private Sub MenuItem_Click(sender As Object, e As RoutedEventArgs)
Dim fileDialog = New OpenFileDialog()
fileDialog.Filter = "PDF files (*.pdf)|*.pdf"
If (fileDialog.ShowDialog() = True) Then
Using document = PdfDocument.Load(fileDialog.FileName)
Me.ImageControl.Source = document.ConvertToImageSource(SaveOptions.Image)
End Using
End If
End Sub
End Class