Performance metrics with large PowerPoint files in C# and VB.NET
GemBox.Presentation is a PowerPoint component that follows .NET design guidelines and best practices. It represents PowerPoint files in-memory through its rich content model that contains slides, frames, shapes, drawings, etc. It has optimized memory consumption, allocation, while not jeopardizing the efficiency and speed of the execution.
The following example shows how you can use BenchmarkDotNet to track the performance of GemBox.Presentation using the provided input PowerPoint file with 15 slides of various content. The file should cover any typical PowerPoint requirements; it includes different kinds of elements (like shapes, images, and tables) and PowerPoint features (like notes, media, and actions).
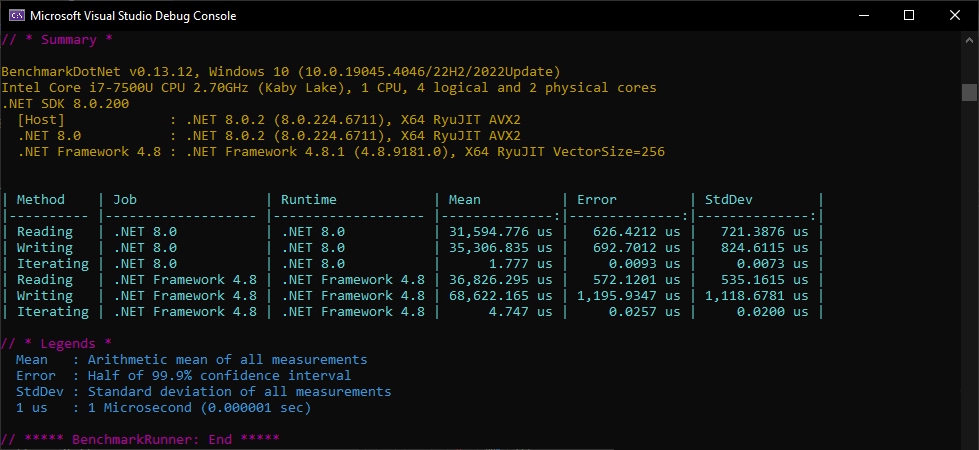
using System.Collections.Generic;
using System.IO;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Engines;
using BenchmarkDotNet.Jobs;
using BenchmarkDotNet.Running;
using GemBox.Presentation;
[SimpleJob(RuntimeMoniker.Net80)]
[SimpleJob(RuntimeMoniker.Net48)]
public class Program
{
private PresentationDocument presentation;
private readonly Consumer consumer = new Consumer();
public static void Main()
{
BenchmarkRunner.Run<Program>();
}
[GlobalSetup]
public void SetLicense()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
// If using Free version and example exceeds its limitations, use Trial or Time Limited version:
// https://www.gemboxsoftware.com/presentation/examples/free-trial-professional/901
this.presentation = PresentationDocument.Load("RandomSlides.pptx");
}
[Benchmark]
public PresentationDocument Reading()
{
return PresentationDocument.Load("RandomSlides.pptx");
}
[Benchmark]
public void Writing()
{
using (var stream = new MemoryStream())
this.presentation.Save(stream, new PptxSaveOptions());
}
[Benchmark]
public void Iterating()
{
this.LoopThroughAllDrawings().Consume(this.consumer);
}
public IEnumerable<Drawing> LoopThroughAllDrawings()
{
foreach (var slide in this.presentation.Slides)
foreach (var drawing in slide.Content.Drawings.All())
yield return drawing;
}
}
Imports System.Collections.Generic
Imports System.IO
Imports BenchmarkDotNet.Attributes
Imports BenchmarkDotNet.Engines
Imports BenchmarkDotNet.Jobs
Imports BenchmarkDotNet.Running
Imports GemBox.Presentation
<SimpleJob(RuntimeMoniker.Net80)>
<SimpleJob(RuntimeMoniker.Net48)>
Public Class Program
Private presentation As PresentationDocument
Private ReadOnly consumer As Consumer = New Consumer()
Public Shared Sub Main()
BenchmarkRunner.Run(Of Program)()
End Sub
<GlobalSetup>
Public Sub SetLicense()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
' If using Free version and example exceeds its limitations, use Trial or Time Limited version:
' https://www.gemboxsoftware.com/presentation/examples/free-trial-professional/901
Me.presentation = PresentationDocument.Load("RandomSlides.pptx")
End Sub
<Benchmark>
Public Function Reading() As PresentationDocument
Return PresentationDocument.Load("RandomSlides.pptx")
End Function
<Benchmark>
Public Sub Writing()
Using stream = New MemoryStream()
Me.presentation.Save(stream, New PptxSaveOptions())
End Using
End Sub
<Benchmark>
Public Sub Iterating()
Me.LoopThroughAllDrawings().Consume(Me.consumer)
End Sub
Public Iterator Function LoopThroughAllDrawings() As IEnumerable(Of Object)
For Each slide In Me.presentation.Slides
For Each drawing In slide.Content.Drawings.All()
Yield drawing
Next
Next
End Function
End Class
Benchmarks for 10,000 PowerPoint slides
The more content you have, the more memory you'll need. The amount of content you can handle depends on a few factors, like the machine's available memory, the application's architecture (32-bit or 64-bit), the targeted .NET platform (.NET Core or .NET Framework), etc.
The following benchmark chart provide the results of working with PowerPoint files with up to 10 thousand slides. It shows a steady and linear increase in time with an increased number of slides. For more information, see the resulting performance measurements in the 10_Thousand_Slides_Performance.xlsx file.
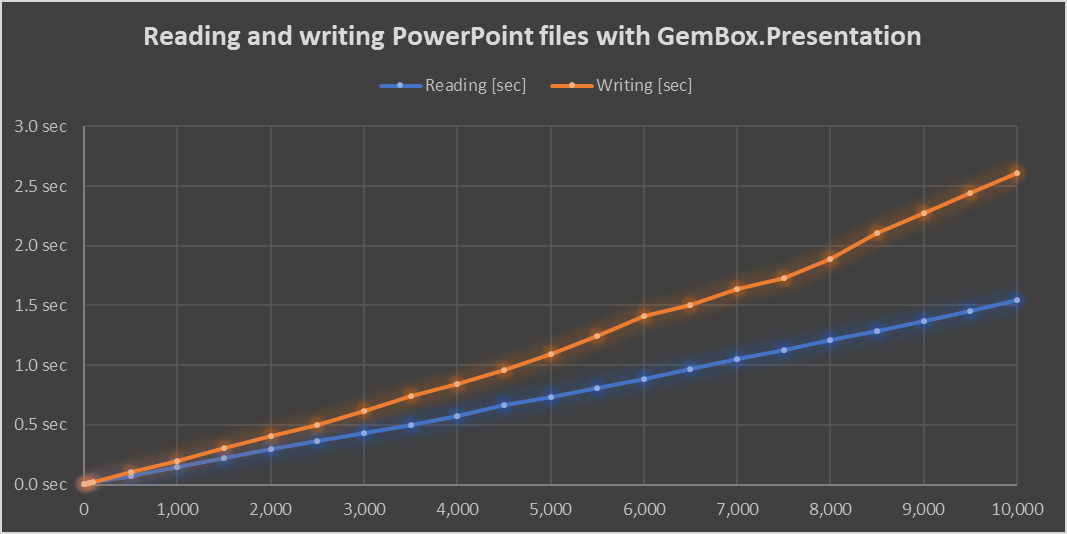