Use PowerPoint slide notes in C# and VB.NET
You can use PowerPoint slide notes in your presentations to provide references and key information about the content. It’s possible to add notes in each slide of a presentation or just in the ones they need to recall about a specific topic.
By default, when you add speaker notes to a presentation slide, it automatically contains several placeholder shapes that are inherited from the MasterNotesSlide
. The placeholder shape with placeholder type PlaceholderType.Text
is the shape that contains notes text (paragraphs).
For more information about notes slides and master notes slide, see the GemBox.Presentation content model help page.
The following example shows how to add notes to a slide using the GemBox.Presentation API, in both C# and VB.NET applications.
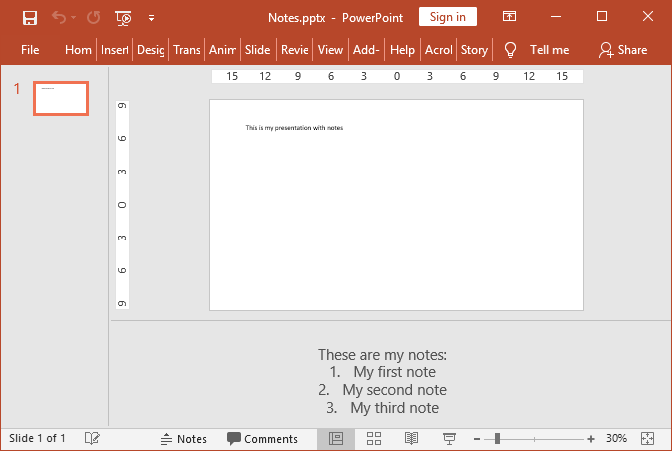
using System.Linq;
using GemBox.Presentation;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
// Add a blank slide to the presentation.
var slide = presentation.Slides.AddNew(SlideLayoutType.Blank);
// Add a text box with some content to the slide.
var text = slide.Content.AddTextBox(3, 2, 22, 2, LengthUnit.Centimeter);
text.AddParagraph().AddRun("This is my presentation with notes");
// Add notes to the slide.
var notes = slide.AddNotes();
// Using LINQ, find shape that contains notes text.
var notesText = notes.Content.Drawings.OfType<Shape>().
Single(sp => sp.Placeholder.PlaceholderType == PlaceholderType.Text).Text;
// Start adding notes text and formatting.
notesText.AddParagraph().AddRun("These are my notes:");
var noteParagraph = notesText.AddParagraph();
// Paragraph will be numbered.
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod;
// Move paragraph content a little bit from its number.
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter);
noteParagraph.AddRun("My first note");
noteParagraph = notesText.AddParagraph();
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod;
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter);
noteParagraph.AddRun("My second note");
noteParagraph = notesText.AddParagraph();
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod;
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter);
noteParagraph.AddRun("My third note");
presentation.Save("Notes.pptx");
}
}
Imports System.Linq
Imports GemBox.Presentation
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument
' Add a blank slide to the presentation.
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Blank)
' Add a text box with some content to the slide.
Dim text = slide.Content.AddTextBox(3, 2, 22, 2, LengthUnit.Centimeter)
text.AddParagraph().AddRun("This is my presentation with notes")
' Add notes to the slide.
Dim notes = slide.AddNotes()
' Using LINQ, find shape that contains notes text.
Dim notesText = notes.Content.Drawings.OfType(Of Shape)().
Single(Function(sp) sp.Placeholder.PlaceholderType = PlaceholderType.Text).Text
' Start adding notes text and formatting.
notesText.AddParagraph().AddRun("These are my notes:")
Dim noteParagraph = notesText.AddParagraph()
' Paragraph will be numbered.
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod
' Move paragraph content a little bit from its number.
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter)
noteParagraph.AddRun("My first note")
noteParagraph = notesText.AddParagraph()
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter)
noteParagraph.AddRun("My second note")
noteParagraph = notesText.AddParagraph()
noteParagraph.Format.List.NumberType = ListNumberType.DecimalPeriod
noteParagraph.Format.IndentationSpecial =
Length.From(-0.64, LengthUnit.Centimeter)
noteParagraph.AddRun("My third note")
presentation.Save("Notes.pptx")
End Sub
End Module