Create PowerPoint file in Xamarin
The following example shows how you can create a PowerPoint file in Xamarin.Forms mobile application using GemBox.Presentation.
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="MainPage">
<StackLayout Padding="50"
Spacing="20">
<Label Text="GemBox.Presentation Example"
HorizontalOptions="Center"
FontSize="Large"
Margin="0,0,0,30" />
<Editor x:Name="text"
VerticalOptions="FillAndExpand"
Text="Hi! This presentation was generated with GemBox.Presentation library." />
<ActivityIndicator x:Name="activity" />
<Button x:Name="button"
Text="Create presentation"
Clicked="Button_Clicked"/>
</StackLayout>
</ContentPage>
using GemBox.Presentation;
using System;
using System.ComponentModel;
using System.IO;
using System.Threading.Tasks;
using Xamarin.Essentials;
using Xamarin.Forms;
public partial class MainPage : ContentPage
{
public MainPage()
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
InitializeComponent();
}
private string CreatePresentation()
{
var presentation = new PresentationDocument();
presentation.Slides.AddNew(SlideLayoutType.Custom)
.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 10, 4, LengthUnit.Centimeter)
.AddParagraph()
.AddRun(text.Text);
var filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments), "Example.pptx");
presentation.Save(filePath);
return filePath;
}
private async void Button_Clicked(object sender, EventArgs e)
{
button.IsEnabled = false;
activity.IsRunning = true;
try
{
var filePath = await Task.Run(() => CreatePresentation());
await Launcher.OpenAsync(new OpenFileRequest(Path.GetFileName(filePath), new ReadOnlyFile(filePath)));
}
catch (Exception ex)
{
await DisplayAlert("Error", ex.Message, "Close");
}
activity.IsRunning = false;
button.IsEnabled = true;
}
}
Imports GemBox.Presentation
Imports System
Imports System.ComponentModel
Imports System.IO
Imports System.Threading.Tasks
Imports Xamarin.Essentials
Imports Xamarin.Forms
Partial Public Class MainPage
Inherits ContentPage
Public Sub New()
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
InitializeComponent()
End Sub
Private Function CreatePresentation() As String
Dim presentation As New PresentationDocument()
presentation.Slides.AddNew(SlideLayoutType.Custom)
.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 10, 4, LengthUnit.Centimeter)
.AddParagraph()
.AddRun(Text.Text)
Dim filePath = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments), "Example.pptx")
presentation.Save(filePath)
Return filePath
End Function
Private Async Sub Button_Clicked(sender As Object, e As EventArgs)
button.IsEnabled = False
activity.IsRunning = True
Try
Dim filePath = Await Task.Run(Function() CreatePresentation())
Await Launcher.OpenAsync(New OpenFileRequest(Path.GetFileName(filePath), New ReadOnlyFile(filePath)))
Catch ex As Exception
Await DisplayAlert("Error", ex.Message, "Close")
End Try
activity.IsRunning = False
button.IsEnabled = True
End Sub
End Class
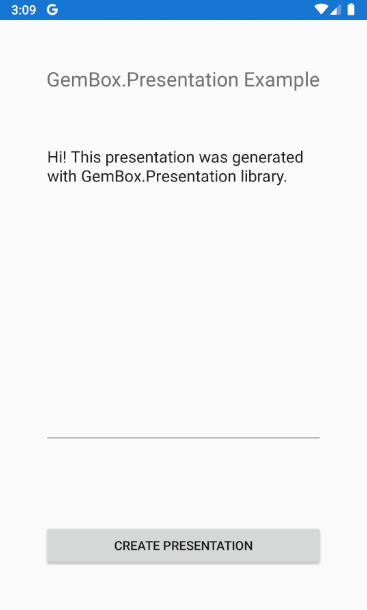
Using full functionality of GemBox.Presentation in Xamarin application requires adjustments explained in detail on Supported Platforms help page.