Create PowerPoint tables
With the example below, you will learn how to use GemBox.Presentation to create and populate a simple table using the GemBox.Presentation API.
using GemBox.Presentation;
using GemBox.Presentation.Tables;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
// Create new presentation slide.
var slide = presentation.Slides.AddNew(SlideLayoutType.Custom);
// Create new table.
var table = slide.Content.AddTable(5, 5, 20, 12, LengthUnit.Centimeter);
// Format table with no-style grid.
table.Format.Style = presentation.TableStyles.GetOrAdd(
TableStyleName.NoStyleTableGrid);
int columnCount = 4;
int rowCount = 10;
for (int i = 0; i < columnCount; i++)
// Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter));
for (int i = 0; i < rowCount; i++)
{
// Create new table row.
var row = table.Rows.AddNew(
Length.From(1.2, LengthUnit.Centimeter));
for (int j = 0; j < columnCount; j++)
{
// Create new table cell.
var cell = row.Cells.AddNew();
// Set table cell text.
cell.Text.AddParagraph().AddRun(
string.Format(null, "Cell {0}-{1}", i + 1, j + 1));
}
}
presentation.Save("Simple Table.%OutputFileType%");
}
}
Imports GemBox.Presentation
Imports GemBox.Presentation.Tables
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument
' Create new presentation slide.
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Custom)
' Create new table.
Dim table = slide.Content.AddTable(5, 5, 20, 12, LengthUnit.Centimeter)
' Format table with no-style grid.
table.Format.Style = presentation.TableStyles.GetOrAdd(
TableStyleName.NoStyleTableGrid)
Dim columnCount As Integer = 4
Dim rowCount As Integer = 10
For i As Integer = 0 To columnCount - 1
' Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter))
Next
For i As Integer = 0 To rowCount - 1
' Create new table row.
Dim row = table.Rows.AddNew(
Length.From(1.2, LengthUnit.Centimeter))
For j As Integer = 0 To columnCount - 1
' Create new table cell.
Dim cell = row.Cells.AddNew()
' Set table cell text.
cell.Text.AddParagraph().AddRun(
String.Format(Nothing, "Cell {0}-{1}", i + 1, j + 1))
Next
Next
presentation.Save("Simple Table.%OutputFileType%")
End Sub
End Module
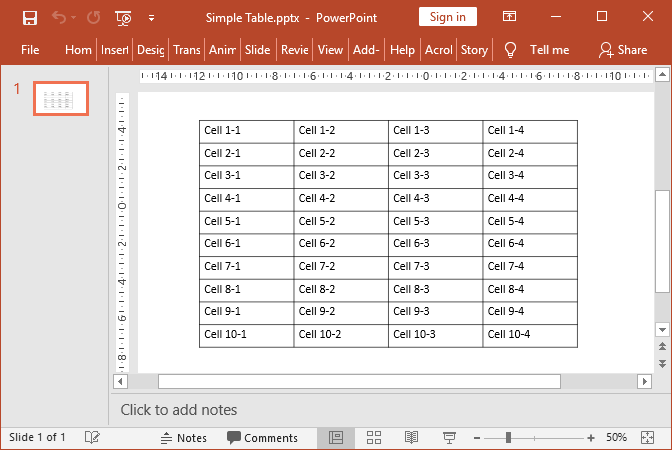
Tables and other content, such as charts or SmartArt diagrams, are embedded in a special kind of drawing, called a The following example shows how you can use the GemBox.Presentation component to create table styles and assign them to tables.GraphicFrame
, contained in the SlideObject.Content
.Set PowerPoint table styles
using GemBox.Presentation;
using GemBox.Presentation.Tables;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
// Create new presentation slide.
var slide = presentation.Slides.AddNew(SlideLayoutType.Custom);
int columnCount = 4;
int rowCount = 5;
// Create new table.
var table = slide.Content.AddTable(1, 1, 20, 4, LengthUnit.Centimeter);
for (int i = 0; i < columnCount; i++)
// Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter));
for (int i = 0; i < rowCount; i++)
{
// Create new table row.
var row = table.Rows.AddNew(
Length.From(8, LengthUnit.Millimeter));
for (int j = 0; j < columnCount; j++)
// Create new table cell.
row.Cells.AddNew().Text.AddParagraph().AddRun(
string.Format(null, "Cell {0}-{1}", i + 1, j + 1));
}
// Set table style.
table.Format.Style = presentation.TableStyles.GetOrAdd(
TableStyleName.MediumStyle2Accent2);
// Set table style options.
table.Format.StyleOptions = TableStyleOptions.FirstRow
| TableStyleOptions.LastRow
| TableStyleOptions.BandedRows;
// Create new table style.
var myStyle = presentation.TableStyles.Create("My Table Styles");
// Get "WholeTable" part style.
var partStyle = myStyle[TablePartStyleType.WholeTable];
// Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.LightGray));
// Get table border style.
var borderStyle = partStyle.Borders;
// Get "InsideHorizontal" border format.
var border = borderStyle[TableCellBorderType.InsideHorizontal];
// Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
border.Width = Length.From(2, LengthUnit.Millimeter);
// Get "InsideVertical" border format.
border = borderStyle[TableCellBorderType.InsideVertical];
// Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
border.Width = Length.From(2, LengthUnit.Millimeter);
// Get "FirstRow" part style.
partStyle = myStyle[TablePartStyleType.FirstRow];
// Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.White));
// Get table border style.
borderStyle = partStyle.Borders;
// Get "Top" border format.
border = borderStyle[TableCellBorderType.Top];
// Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.Black));
border.Width = Length.From(2, LengthUnit.Millimeter);
// Get "Bottom" border format.
border = borderStyle[TableCellBorderType.Bottom];
// Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.Black));
border.Width = Length.From(2, LengthUnit.Millimeter);
// Get table text style.
var textStyle = partStyle.Text;
// Set text format.
textStyle.Bold = true;
textStyle.Color = Color.FromName(ColorName.DarkGray);
// Get "LastRow" part style.
partStyle = myStyle[TablePartStyleType.LastRow];
// Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.White));
// Get table border style.
borderStyle = partStyle.Borders;
// Set "Top" border line format.
borderStyle[TableCellBorderType.Top].Fill.SetSolid(
Color.FromName(ColorName.Black));
borderStyle[TableCellBorderType.Top].Width =
Length.From(2, LengthUnit.Millimeter);
// Set "Bottom" border line format.
borderStyle[TableCellBorderType.Bottom].Fill.SetSolid(
Color.FromName(ColorName.Black));
borderStyle[TableCellBorderType.Bottom].Width =
Length.From(2, LengthUnit.Millimeter);
// Set text format.
partStyle.Text.Bold = true;
partStyle.Text.Color = Color.FromName(ColorName.DarkGray);
// Create new table.
table = slide.Content.AddTable(1, 8, 20, 4, LengthUnit.Centimeter);
for (int i = 0; i < columnCount; i++)
// Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter));
for (int i = 0; i < rowCount; i++)
{
// Create new table row.
var row = table.Rows.AddNew(
Length.From(8, LengthUnit.Millimeter));
for (int j = 0; j < columnCount; j++)
// Create new table cell.
row.Cells.AddNew().Text.AddParagraph().AddRun(
string.Format(null, "Cell {0}-{1}", i + 1, j + 1));
}
// Set table style.
table.Format.Style = myStyle;
// Set table style options.
table.Format.StyleOptions = TableStyleOptions.FirstRow
| TableStyleOptions.LastRow;
presentation.Save("Table Styles.%OutputFileType%");
}
}
Imports GemBox.Presentation
Imports GemBox.Presentation.Tables
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument
' Create new presentation slide.
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Custom)
Dim columnCount As Integer = 4
Dim rowCount As Integer = 5
' Create new table.
Dim table = slide.Content.AddTable(1, 1, 20, 4, LengthUnit.Centimeter)
For i As Integer = 0 To columnCount - 1
' Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter))
Next
For i As Integer = 0 To rowCount - 1
' Create new table row.
Dim row = table.Rows.AddNew(
Length.From(8, LengthUnit.Millimeter))
For j As Integer = 0 To columnCount - 1
' Create new table cell.
row.Cells.AddNew().Text.AddParagraph().AddRun(
String.Format(Nothing, "Cell {0}-{1}", i + 1, j + 1))
Next
Next
' Set table style.
table.Format.Style = presentation.TableStyles.GetOrAdd(
TableStyleName.MediumStyle2Accent2)
' Set table style options.
table.Format.StyleOptions = TableStyleOptions.FirstRow Or
TableStyleOptions.LastRow Or
TableStyleOptions.BandedRows
' Create New table style.
Dim myStyle = presentation.TableStyles.Create("My Table Styles")
' Get "WholeTable" part style.
Dim partStyle = myStyle(TablePartStyleType.WholeTable)
' Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.LightGray))
' Get table border style.
Dim borderStyle = partStyle.Borders
' Get "InsideHorizontal" border format.
Dim border = borderStyle(TableCellBorderType.InsideHorizontal)
' Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
border.Width = Length.From(2, LengthUnit.Millimeter)
' Get "InsideVertical" border format.
border = borderStyle(TableCellBorderType.InsideVertical)
' Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
border.Width = Length.From(2, LengthUnit.Millimeter)
' Get "FirstRow" part style.
partStyle = myStyle(TablePartStyleType.FirstRow)
' Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.White))
' Get table border style.
borderStyle = partStyle.Borders
' Get "Top" border format.
border = borderStyle(TableCellBorderType.Top)
' Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.Black))
border.Width = Length.From(2, LengthUnit.Millimeter)
' Get "Bottom" border format.
border = borderStyle(TableCellBorderType.Bottom)
' Set border line format.
border.Fill.SetSolid(Color.FromName(ColorName.Black))
border.Width = Length.From(2, LengthUnit.Millimeter)
' Get table text style.
Dim textStyle = partStyle.Text
' Set text format.
textStyle.Bold = True
textStyle.Color = Color.FromName(ColorName.DarkGray)
' Get "LastRow" part style.
partStyle = myStyle(TablePartStyleType.LastRow)
' Set fill format.
partStyle.Fill.SetSolid(Color.FromName(ColorName.White))
' Get table border style.
borderStyle = partStyle.Borders
' Set "Top" border line format.
borderStyle(TableCellBorderType.Top).Fill.SetSolid(
Color.FromName(ColorName.Black))
borderStyle(TableCellBorderType.Top).Width =
Length.From(2, LengthUnit.Millimeter)
' Set "Bottom" border line format.
borderStyle(TableCellBorderType.Bottom).Fill.SetSolid(
Color.FromName(ColorName.Black))
borderStyle(TableCellBorderType.Bottom).Width =
Length.From(2, LengthUnit.Millimeter)
' Set text format.
partStyle.Text.Bold = True
partStyle.Text.Color = Color.FromName(ColorName.DarkGray)
' Create new table.
table = slide.Content.AddTable(1, 8, 20, 4, LengthUnit.Centimeter)
For i As Integer = 0 To columnCount - 1
' Create new table column.
table.Columns.AddNew(Length.From(5, LengthUnit.Centimeter))
Next
For i As Integer = 0 To rowCount - 1
' Create new table row.
Dim row = table.Rows.AddNew(
Length.From(8, LengthUnit.Millimeter))
For j As Integer = 0 To columnCount - 1
' Create new table cell.
row.Cells.AddNew().Text.AddParagraph().AddRun(
String.Format(Nothing, "Cell {0}-{1}", i + 1, j + 1))
Next
Next
' Set table style.
table.Format.Style = myStyle
' Set table style options.
table.Format.StyleOptions = TableStyleOptions.FirstRow Or
TableStyleOptions.LastRow
presentation.Save("Table Styles.%OutputFileType%")
End Sub
End Module