Export PowerPoint in WPF
With the following example, you can learn how to use GemBox.Presentation to convert a PowerPoint presentation to an XpsDocument
instance and attach it to WPF's DocumentViewer
control.
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Export to XpsDocument Example"
SizeToContent="WidthAndHeight">
<DocumentViewer x:Name="DocumentViewer"/>
</Window>
using GemBox.Presentation;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Xps.Packaging;
public partial class MainWindow : Window
{
XpsDocument xpsDocument;
public MainWindow()
{
InitializeComponent();
this.SetDocumentViewer(this.DocumentViewer);
}
private void SetDocumentViewer(DocumentViewer documentViewer)
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
var slide = presentation.Slides.AddNew(SlideLayoutType.Custom);
var textBox = slide.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 8, 4, LengthUnit.Centimeter);
textBox.Shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
var run = textBox.AddParagraph().AddRun("Hello World!");
run.Format.Fill.SetSolid(Color.FromName(ColorName.Black));
// XpsDocument needs to stay referenced so that DocumentViewer can access additional required resources.
// Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will not work.
this.xpsDocument = presentation.ConvertToXpsDocument(SaveOptions.Xps);
documentViewer.Document = this.xpsDocument.GetFixedDocumentSequence();
}
}
Imports GemBox.Presentation
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Xps.Packaging
Class MainWindow
Dim xpsDoc As XpsDocument
Public Sub New()
InitializeComponent()
Me.SetDocumentViewer(Me.DocumentViewer)
End Sub
Private Sub SetDocumentViewer(documentViewer As DocumentViewer)
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument()
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Custom)
Dim textBox = slide.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 8, 4, LengthUnit.Centimeter)
textBox.Shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
Dim run = textBox.AddParagraph().AddRun("Hello World!")
run.Format.Fill.SetSolid(Color.FromName(ColorName.Black))
' XpsDocument needs to stay referenced so that DocumentViewer can access additional required resources.
' Otherwise, GC will collect/dispose XpsDocument and DocumentViewer will not work.
Me.xpsDoc = presentation.ConvertToXpsDocument(SaveOptions.Xps)
documentViewer.Document = Me.xpsDoc.GetFixedDocumentSequence()
End Sub
End Class
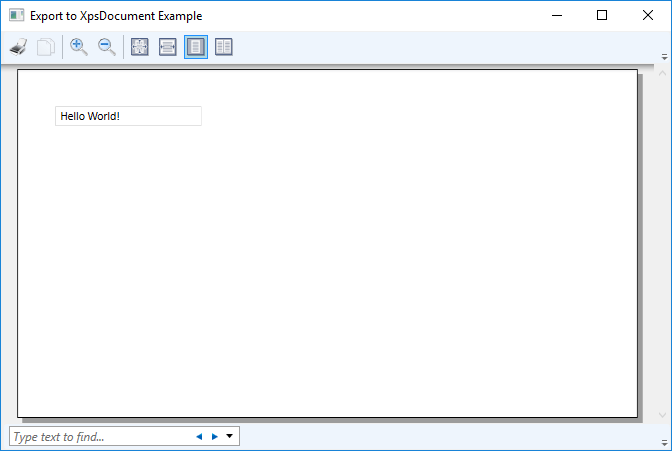
Export PowerPoint to ImageSource in WPF
The following example shows how to use GemBox.Presentation to convert a presentation slide to an ImageSource instance and attach the converted image to WPF's Image control.
<Window x:Class="MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Export to ImageSource Example"
SizeToContent="WidthAndHeight">
<Border Margin="10" BorderBrush="Black" BorderThickness="1">
<Image x:Name="ImageControl"/>
</Border>
</Window>
using GemBox.Presentation;
using System.Windows;
using System.Windows.Controls;
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
SetImageSource(this.ImageControl);
}
private static void SetImageSource(Image image)
{
ComponentInfo.SetLicense("FREE-LIMITED-KEY");
var presentation = new PresentationDocument();
var slide = presentation.Slides.AddNew(SlideLayoutType.Custom);
var textBox = slide.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 8, 4, LengthUnit.Centimeter);
textBox.Shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray));
var run = textBox.AddParagraph().AddRun("Hello World!");
run.Format.Fill.SetSolid(Color.FromName(ColorName.Black));
image.Source = presentation.ConvertToImageSource(SaveOptions.Image);
}
}
Imports GemBox.Presentation
Imports System.Windows
Imports System.Windows.Controls
Class MainWindow
Public Sub New()
InitializeComponent()
SetImageSource(Me.ImageControl)
End Sub
Private Shared Sub SetImageSource(image As Image)
ComponentInfo.SetLicense("FREE-LIMITED-KEY")
Dim presentation = New PresentationDocument()
Dim slide = presentation.Slides.AddNew(SlideLayoutType.Custom)
Dim textBox = slide.Content.AddTextBox(ShapeGeometryType.Rectangle, 2, 2, 8, 4, LengthUnit.Centimeter)
textBox.Shape.Format.Outline.Fill.SetSolid(Color.FromName(ColorName.DarkGray))
Dim run = textBox.AddParagraph().AddRun("Hello World!")
run.Format.Fill.SetSolid(Color.FromName(ColorName.Black))
image.Source = presentation.ConvertToImageSource(SaveOptions.Image)
End Sub
End Class
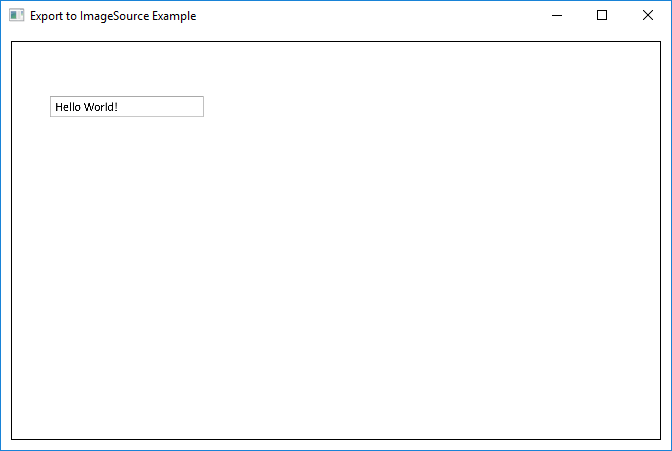
The WPF Image control allows you to display images in an application. You can use the GemBox.Presentation API to convert a PowerPoint slide to an image.