Convert Excel files to PDF
The following example shows how to convert an Excel file to PDF in C# and VB.NET using the GemBox.Spreadsheet library.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// In order to convert Excel to PDF, we just need to:
// 1. Load XLS or XLSX file into ExcelFile object.
// 2. Save ExcelFile object to PDF file.
ExcelFile workbook = ExcelFile.Load("%InputFileName%");
workbook.Save("Convert.pdf", new PdfSaveOptions() { SelectionType = SelectionType.EntireFile });
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' In order to convert Excel to PDF, we just need to
' 1. Load XLS Or XLSX file into ExcelFile object.
' 2. Save ExcelFile object to PDF file.
Dim workbook As ExcelFile = ExcelFile.Load("%InputFileName%")
workbook.Save("Convert.pdf", New PdfSaveOptions() With {.SelectionType = SelectionType.EntireFile})
End Sub
End Module
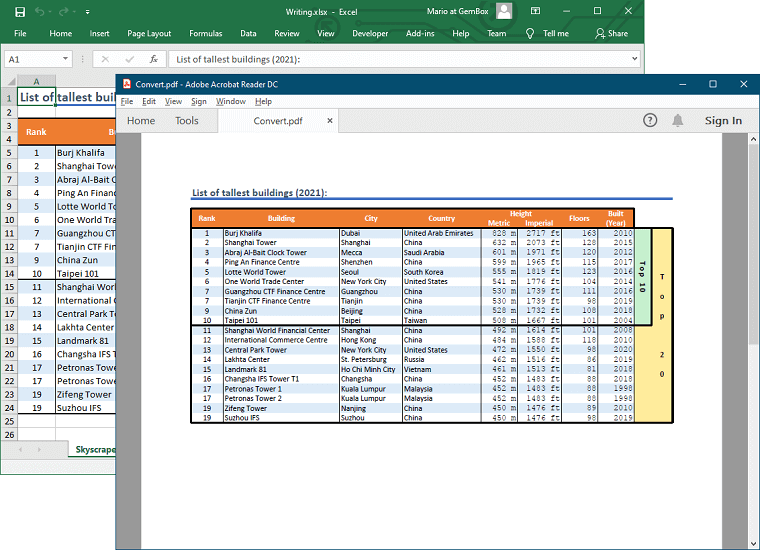
Note that when converting an Excel workbook to PDF, the machine that's executing the code should have the fonts that are used in the workbook installed on it. If not, you can provide them as custom fonts. In addition to saving the whole Excel workbook as a single PDF file, you can also convert each sheet into a separate PDF file, as shown in the example below. GemBox.Spreadsheet also supports saving the Excel file to PDF/A, an ISO-standardized version of the Portable Document Format (PDF) specialized for use in the archiving and long-term preservation of electronic documents. The following example shows how you can convert an Excel file to PDF/A using Convert Excel range to PDF
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
// Load Excel file.
ExcelFile workbook = ExcelFile.Load("%InputFileName%");
// Get Excel sheet you want to export.
ExcelWorksheet worksheet = workbook.Worksheets[0];
// Set targeted sheet as active.
workbook.Worksheets.ActiveWorksheet = worksheet;
// Get cell range that you want to export.
CellRange range = worksheet.Cells.GetSubrange("A5:I14");
// Set targeted range as print area.
worksheet.NamedRanges.SetPrintArea(range);
// Save to PDF file.
// By default, the SelectionType.ActiveSheet is used.
workbook.Save("ConvertRange.pdf");
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
' Load Excel file.
Dim workbook As ExcelFile = ExcelFile.Load("%InputFileName%")
' Get Excel sheet you want to export.
Dim worksheet As ExcelWorksheet = workbook.Worksheets(0)
' Set targeted sheet as active.
workbook.Worksheets.ActiveWorksheet = worksheet
' Get cell range that you want to export.
Dim range As CellRange = worksheet.Cells.GetSubrange("A5:I14")
' Set targeted range as print area.
worksheet.NamedRanges.SetPrintArea(range)
' Save to PDF file.
' By default, the SelectionType.ActiveSheet is used.
workbook.Save("ConvertRange.pdf")
End Sub
End Module
Convert Excel files to PDF/A
PdfConformanceLevel
to specify the conformance level and version.using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
PdfConformanceLevel conformanceLevel = %PdfConformanceLevel%;
// Load Excel file.
var workbook = ExcelFile.Load("%InputFileName%");
// Create PDF save options.
var options = new PdfSaveOptions()
{
ConformanceLevel = conformanceLevel
};
// Save to PDF file.
workbook.Save("ConvertWithConformance.pdf", options);
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim conformanceLevel As PdfConformanceLevel = %PdfConformanceLevel%
' Load Excel file.
Dim workbook = ExcelFile.Load("%InputFileName%")
' Create PDF save options.
Dim options As New PdfSaveOptions() With
{
.ConformanceLevel = conformanceLevel
}
' Save to PDF file.
workbook.Save("ConvertWithConformance.pdf", options)
End Sub
End Module