Add Hyperlinks to Excel
The following example shows how to add hyperlinks to Excel cells with URL (external) or cell (internal) as their destination, using GemBox.Spreadsheet.
using GemBox.Spreadsheet;
class Program
{
static void Main()
{
// If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
var workbook = new ExcelFile();
var worksheet = workbook.Worksheets.Add("Hyperlinks");
var hyperlinkStyle = workbook.Styles[BuiltInCellStyleName.Hyperlink];
var cell = worksheet.Cells["B1"];
cell.Value = "Link to GemBox homepage";
cell.Style = hyperlinkStyle;
cell.Hyperlink.Location = "https://www.gemboxsoftware.com";
cell.Hyperlink.IsExternal = true;
cell = worksheet.Cells["B3"];
cell.Value = "Jump";
cell.Style = hyperlinkStyle;
cell.Hyperlink.ToolTip = "This is tool tip! This hyperlink jumps to E1.";
cell.Hyperlink.Location = worksheet.Name + "!E3";
worksheet.Cells["E3"].Value = "Jump destination";
cell = worksheet.Cells["B5"];
cell.Formula = "=HYPERLINK(\"https://www.gemboxsoftware.com/spreadsheet/examples/excel-cell-hyperlinks/207\", \"Link to Hyperlinks example\")";
cell.Style = hyperlinkStyle;
workbook.Save("Hyperlinks.%OutputFileType%");
}
}
Imports GemBox.Spreadsheet
Module Program
Sub Main()
' If using the Professional version, put your serial key below.
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY")
Dim workbook As New ExcelFile()
Dim worksheet = workbook.Worksheets.Add("Hyperlinks")
Dim hyperlinkStyle = workbook.Styles(BuiltInCellStyleName.Hyperlink)
Dim cell = worksheet.Cells("B1")
cell.Value = "Link to GemBox homepage"
cell.Style = hyperlinkStyle
cell.Hyperlink.Location = "https://www.gemboxsoftware.com"
cell.Hyperlink.IsExternal = True
cell = worksheet.Cells("B3")
cell.Value = "Jump"
cell.Style = hyperlinkStyle
cell.Hyperlink.ToolTip = "This is tool tip! This hyperlink jumps to E1."
cell.Hyperlink.Location = worksheet.Name + "!E3"
worksheet.Cells("E3").Value = "Jump destination"
cell = worksheet.Cells("B5")
cell.Formula = "=HYPERLINK(""https://www.gemboxsoftware.com/spreadsheet/examples/excel-cell-hyperlinks/207"", ""Link to Hyperlinks example"")"
cell.Style = hyperlinkStyle
workbook.Save("Hyperlinks.%OutputFileType%")
End Sub
End Module
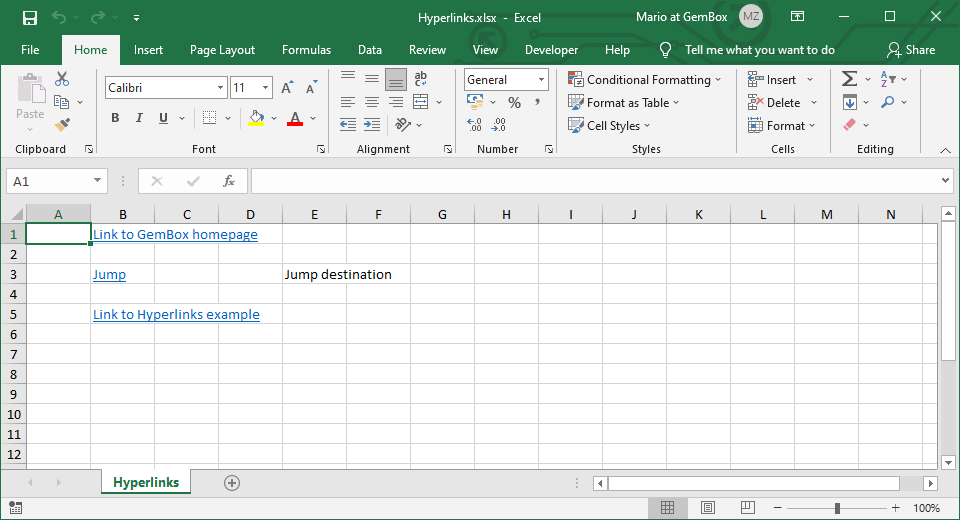
You can use hyperlinks to link to external resources (URLs) or cells. To remove a hyperlink, just set this property to null
. You can also retrieve or delete any hyperlink within a worksheet using the ExcelWorksheet.Hyperlinks
collection.
Besides the Hyperlink
property, you can also use the HYPERLINK formula to create a hyperlink, but note:
- In ODS files, HYPERLINK and other OpenOffice formulas use a semicolon as an argument list separator, unlike Excel formulas which use a comma.
- In PDF and HTML files, HYPERLINK and other formulas must be calculated before exporting.